California Iso Download Csv Data
Data Downloads. To download data related to alternative fuels and advanced vehicles, follow the steps below. Choose data to download. Choose the dataset and file format you want to download. File Format Read descriptions of the data included in the alternative. Below are free Area Code quick references in printable format. Each list includes area codes and helpful additional information such as time zones. Area Code / Time Zones This is our most popular list. On a single page, it has each area code with corresponding time zone and state.
PermalinkJoin GitHub today
GitHub is home to over 36 million developers working together to host and review code, manage projects, and build software together.
Sign up# CAISO-oasisAPI-operations.R |
# |
# Contributors: Peter Alstone |
# |
# Version 0.1 |
# |
# These R functions can be used to access CAISO operations data, an alternative to using the 'Oasis' system |
# get R: cran.r-project.org |
# FUNCTIONS ------------------------------------------------ |
# fetch CAISO LMP data from OASIS (see 'Interface Specification for OASIS API) |
getCAISOlmp<-function(startdate=20110101, enddate=20110102, market_run_id='DAM',node='ALL', |
onlyDo=NA,allAtOnce=TRUE){ |
# # DON'T use node by node , only use ALL nodes -- doesn't append data! |
# this function simply grabs the csv data from caiso and dumps it into a data frame with the same structure as the csv. |
# onlyDo means 'only do the first X nodes in the list' |
# allAtOnce means to use the 'all apnode' command to download instead of node by node crawling -- use anything else at your peril. |
# convert CAISO format to POSIXct dates |
start<- strptime(startdate,'%Y%m%d') |
end<- strptime(enddate,'%Y%m%d') |
# Initialize data frame with starting day |
activeDay<-start |
#define base URL |
baseURL<-'http://oasis.caiso.com/mrtu-oasis/SingleZip?' |
if(node!='ALL'){ #if there is only one node...just do that. |
while(activeDay<end){ |
activeNode<-node |
# assemble url for LMP |
getURL<- paste(baseURL,'resultformat=6&queryname=PRC_LMP&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),'&market_run_id=',market_run_id,'&node=',activeNode,sep='') |
temp<- tempfile() #create temp file |
download.file(getURL,temp) # get data into temp |
data<- read.csv(unzip(temp)) # unzip and read csv, dump into data |
unlink(temp) |
#end for |
activeDay<-activeDay+86400# add one day (in seconds) |
#end while |
} |
return(data) |
}else{ #...or get all of the nodes... |
if(allAtOnce){ #get all nodes day by day. |
# First day -- initialize data output frame. |
getURL<- paste(baseURL,'resultformat=6&queryname=PRC_LMP&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),'&market_run_id=',market_run_id,'&grp_type=ALL_APNODES',sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(data<- read.csv(unzip(temp))) # unzip and read csv, dump into data |
unlink(temp) |
activeDay<-activeDay+86400#increment one day |
while(activeDay<end){ |
# assemble url for LMP |
getURL<- paste(baseURL,'resultformat=6&queryname=PRC_LMP&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),'&market_run_id=',market_run_id,'&grp_type=ALL_APNODES',sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(newdata<- read.csv(unzip(temp))) # unzip and read csv, dump into data |
unlink(temp) |
try(data<- rbind(data,newdata))#append new data to existing |
activeDay<-activeDay+86400# add one day (in seconds) |
} |
}else{ # go through pnodes one by one (very slow) |
#get list of all Pnodes (this may or may not be all...just take the start date) |
pnodeURL<- paste(baseURL,'resultformat=6&queryname=ATL_PNODE&Pnode_type=ALL&startdate=',strftime(start,'%Y%m%d'),sep='') |
temp<- tempfile() #create temp file |
download.file(pnodeURL,temp) # get data into temp |
pnode.desc<- read.csv(unzip(temp)) # unzip and read csv, dump into data |
unlink(temp) |
if(is.na(onlyDo)){numberNodes<- length(pnode.desc$PNODE_ID)}else{numberNodes<-onlyDo} |
activeNode<-pnode.desc$PNODE_ID[1] |
# assemble url for LMP and download. |
getURL<- paste(baseURL,'resultformat=6&queryname=PRC_LMP&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),'&market_run_id=',market_run_id,'&node=',activeNode,sep='') |
temp<- tempfile() #create temp file |
download.file(getURL,temp) # get data into temp |
data<- read.csv(unzip(temp)) # unzip and read csv, dump into data (THE MAIN OUTPUT) |
unlink(temp) |
while(activeDay<end){ |
for(iin2:numberNodes){ |
activeNode<-pnode.desc$PNODE_ID[i] |
# assemble url for LMP |
getURL<- paste(baseURL,'resultformat=6&queryname=PRC_LMP&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),'&market_run_id=',market_run_id,'&node=',activeNode,sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(newdata<- read.csv(unzip(temp))) # unzip and read csv, dump into data |
unlink(temp) |
try(data<- rbind(data,newdata))#append new data to existing DF |
} |
#end for |
activeDay<-activeDay+86400# add one day (in seconds) |
} #end while |
} #end else |
return(data) |
} #end else |
} #end FUNCTION ... getCAISOlmp |
# fetch CAISO energy clearing data from OASIS (see 'Interface Specification for OASIS API) |
getCAISOsysenergy<-function(startdate=20110101, enddate=20110102, |
market_run_id=c('DAM','RTM','RUC','HASP')){ |
# this function simply grabs the csv data from caiso and dumps it into a data frame with the same structure as the csv. |
# use a set of market run IDs to get all of them using c(oncatenate)...otherwise specify 'DAM' 'RTM' 'RUC' or 'HASP' |
# convert CAISO format to POSIXct dates |
start<- strptime(startdate,'%Y%m%d') |
end<- strptime(enddate,'%Y%m%d') |
# Initialize data frame with starting day |
activeDay<-start |
#define base URL |
baseURL<-'http://oasis.caiso.com/mrtu-oasis/SingleZip?' |
# First day -- initialize data output frame with first data. |
dummy<-1 |
for(iinmarket_run_id){ |
getURL<- paste(baseURL,'resultformat=6&queryname=ENE_SLRS&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),'&market_run_id=',i,'&tac_zone_name=ALL&schedule=ALL',sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
if(dummy1){try(data<- read.csv(unzip(temp))) |
dummy=dummy+1 |
}else{ |
try(newdata<- read.csv(unzip(temp))) |
try(data<- rbind(data,newdata))#append new data to existing |
} # unzip and read csv, dump into data |
unlink(temp) |
} #end for loop for first day |
activeDay<-activeDay+86400# add one day (in seconds) |
# Subsequent days -- download and append |
while(activeDay<end){ |
for(iinmarket_run_id){ #loop through market types |
getURL<- paste(baseURL,'resultformat=6&queryname=ENE_SLRS&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),'&market_run_id=',i,'&tac_zone_name=ALL&schedule=ALL',sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(newdata<- read.csv(unzip(temp))) # unzip and read csv, dump into data |
unlink(temp) |
try(data<- rbind(data,newdata))#append new data to existing |
} |
activeDay<-activeDay+86400# add one day (in seconds) |
} #end while loop for moving through time... |
return(data) # these are the data you are looking for... |
} #end FUNCTION ... getCAISOsysenergy |
# fetch CAISO load data from OASIS (see 'Interface Specification for OASIS API) |
getCAISOload<-function(startdate=20110101, enddate=20110102){ |
# this function simply grabs the csv data from caiso and dumps it into a data frame with the same structure as the csv. |
# convert CAISO format to POSIXct dates |
start<- strptime(startdate,'%Y%m%d') |
end<- strptime(enddate,'%Y%m%d') |
# Initialize data frame with starting day |
activeDay<-start |
#define base URL |
baseURL<-'http://oasis.caiso.com/mrtu-oasis/SingleZip?' |
# First day -- initialize data output frame with first data. |
getURL<- paste(baseURL,'resultformat=6&queryname=SLD_FCST&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(data<- read.csv(unzip(temp))) |
unlink(temp) |
activeDay<-activeDay+86400# add one day (in seconds) |
# Subsequent days -- download and append |
while(activeDay<end){ |
getURL<- paste(baseURL,'resultformat=6&queryname=SLD_FCST&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(newdata<- read.csv(unzip(temp))) # unzip and read csv, dump into data |
unlink(temp) |
try(data<- rbind(data,newdata))#append new data to existing |
activeDay<-activeDay+86400# add one day (in seconds) |
} #end while loop for moving through time... |
return(data) # these are the data you are looking for... |
} #end FUNCTION ... getCAISOload |
# Fetch CAISO public bid data (be careful, these are big files) |
getCAISObids<-function(startdate=20110101, enddate=20110102, market='DAM'){ |
#market is DAM or RTM |
# this function simply grabs the csv data from caiso and dumps it into a data frame with the same structure as the csv. |
# convert CAISO format to POSIXct dates |
start<- strptime(startdate,'%Y%m%d') |
end<- strptime(enddate,'%Y%m%d') |
# Initialize data frame with starting day |
activeDay<-start |
#define base URL |
baseURL<-'http://oasis.caiso.com/mrtu-oasis/GroupZip?'#note this is a grouped file |
reportname<-paste('PUB_',market,'_GRP',sep='') |
# First day -- initialize data output frame with first data. |
getURL<- paste(baseURL,'resultformat=6&groupid=',reportname,'&startdate=',strftime(activeDay,'%Y%m%d'),sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(data<- read.csv(unzip(temp))) |
unlink(temp) |
activeDay<-activeDay+86400# add one day (in seconds) |
# Subsequent days -- download and append |
while(activeDay<end){ |
getURL<- paste(baseURL,'resultformat=6&groupid=',reportname,'&startdate=',strftime(activeDay,'%Y%m%d'),sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(newdata<- read.csv(unzip(temp))) # unzip and read csv, dump into data |
unlink(temp) |
try(data<- rbind(data,newdata))#append new data to existing |
activeDay<-activeDay+86400# add one day (in seconds) |
} #end while loop for moving through time... |
return(data) # these are the data you are looking for... |
} #end FUNCTION ... getCAISObids |
# Fetch CAISO public bid data (be careful, these are big files) |
getCAISOopres<-function(startdate=20110101, enddate=20110102){ |
#market is DAM or RTM |
# this function simply grabs the csv data from caiso and dumps it into a data frame with the same structure as the csv. |
# convert CAISO format to POSIXct dates |
start<- strptime(startdate,'%Y%m%d') |
end<- strptime(enddate,'%Y%m%d') |
# Initialize data frame with starting day |
activeDay<-start |
#define base URL |
baseURL<-'http://oasis.caiso.com/mrtu-oasis/SingleZip?'#note this is a grouped file |
# First day -- initialize data output frame with first data. |
getURL<- paste(baseURL,'resultformat=6&queryname=AS_OP_RSRV&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(data<- read.csv(unzip(temp))) |
unlink(temp) |
activeDay<-activeDay+86400# add one day (in seconds) |
# Subsequent days -- download and append |
while(activeDay<end){ |
getURL<- paste(baseURL,'resultformat=6&queryname=AS_OP_RSRV&startdate=',strftime(activeDay,'%Y%m%d'),'&enddate=',strftime(activeDay,'%Y%m%d'),sep='') |
temp<- tempfile() #create temp file |
try(download.file(getURL,temp)) # get data into temp |
try(newdata<- read.csv(unzip(temp))) # unzip and read csv, dump into data |
unlink(temp) |
try(data<- rbind(data,newdata))#append new data to existing |
activeDay<-activeDay+86400# add one day (in seconds) |
} #end while loop for moving through time... |
return(data) # these are the data you are looking for... |
} #end FUNCTION ... getCAISOopres |
# add date info to CAISO data frames |
addDatesCAISO<-function(caisodata,date.format=c('%Y%m%d'),date.column='OPR_DT'){ |
caisodata$posixlt<- as.POSIXlt(caisodata[[date.column]]) |
caisodata$year<-caisodata$posixlt$year+1900 |
caisodata$mon<- strftime(caisodata$posixlt,'%b') |
caisodata$monnum<-strftime(caisodata$posixlt,'%m') |
caisodata$mday<-caisodata$posixlt$mday |
caisodata$wday<- strftime(caisodata$posixlt,'%a') #week day |
caisodata$date<- strftime(caisodata$posixlt,date.format) |
caisodata$yhr<-caisodata$posixlt$yday*24+caisodata$posixlt$hour+1#hour ending 1-8760 over the year |
caisodata$yday<-caisodata$posixlt$yday |
return(caisodata) |
} |
# melt CAISO data into a mono-value data frame for analysis. |
meltCAISO<-function(caisodata,preserve.na=FALSE){ |
hourLabels<- c('HE01','HE02','HE03','HE04','HE05','HE06','HE07','HE08','HE09','HE10','HE11','HE12','HE13','HE14','HE15','HE16','HE17','HE18','HE19','HE20','HE21','HE22','HE23','HE24') |
data<- melt(caisodata,id=which(!names(caisodata) %in%hourLabels),measured=which(names(caisodata) %in%hourLabels,na.rm=preserve.na)) |
return(data) |
} |
# Load duration curves with classic R look saved to pdf |
makeLDC<-function(data,value.column,file='mypdf.pdf', |
h=5,w=10, |
xlab='Exceedance (unitless)',ylab='value', |
main='Load Duration Curve',sub='CAISO total service area',type='p'){ |
data<-arrange(data,desc(data[[value.column]])) |
pdf(file,width=w,height=h) |
cumdist<-1:length(data[[value.column]]) / length(data[[value.column]]) |
myplot<-plot(cumdist,data[[value.column]],xlab=xlab,ylab=ylab,main=main,sub=sub,type=type) |
print(myplot) |
dev.off()} |
# Load duration curves with classic R look ready to send to a graphics device. |
viewLDC<-function(data,value.column, |
color, #use same number of colors as value column! |
h=5,w=10, |
xlab='Exceedance (unitless)',ylab='value', |
main='Load Duration Curve',sub='CAISO total service area',type='p'){ |
if(length(value.column)1){ |
# for first value column |
data<-arrange(data,desc(data[[value.column]])) |
cumdist<-1:length(data[[value.column]]) / length(data[[value.column]]) |
myplot<-plot(cumdist,data[[value.column]],col=color[1],xlab=xlab,ylab=ylab,main=main,sub=sub,type=type) |
return(myplot) |
}else{ |
# for first value column initialize plot |
active.data<-arrange(data,desc(data[[value.column[1]]])) |
cumdist<-1:length(active.data[[value.column[1]]]) / length(active.data[[value.column[1]]]) |
myplot<-plot(cumdist,active.data[[value.column[1]]],col=color[1],xlab=xlab,ylab=ylab,main=main,sub=sub,type=type) |
for(iin2:length(value.column)){ |
active.data<-arrange(data,desc(data[[value.column[i]]])) |
cumdist<-1:length(active.data[[value.column[i]]]) / length(active.data[[value.column[i]]]) |
points(cumdist,active.data[[value.column[i]]],col=color[i]) |
} |
return(myplot) |
}}#end function view LDC |
# add temporal categories (useful for slicing up data by season / peak / weekend) |
addTimeCats<-function(data,time.column='posixlt',hour.column='dhr'){ |
begin.summer<-5#first month of summer |
end.summer<-9#last month of summer |
begin.peak.sum<-13# first hour on peak |
end.peak.sum<-19# last hour on peak |
begin.peak.wint<-17 |
end.peak.wint<-20 |
for(iin1:length(data[[time.column]])){ |
month<-data[[time.column]][['mon']][i]+1 |
hour<-data[[hour.column]][i] |
day<-data[[time.column]][['wday']][i] |
season<-if(month<=begin.summer|month>=end.summer){'winter'}else{'summer'} |
daytype<-if(day0|day6){'weekend'}else{'weekday'} |
period<-if(daytype'weekend'){'off.peak'}else{if(season'summer'&begin.peak.sum<=hour&hour<=end.peak.sum){'peak'}else{if(season'winter'&begin.peak.wint<=hour&hour<=end.peak.wint){'peak'}else{'off.peak'}}} |
data$season[i] <-season |
data$period[i] <-period |
data$daytype[i] <-daytype |
} |
return(data) |
} |
# Arrange ggplots (appropriated from http://gettinggeneticsdone.blogspot.com/2010/03/arrange-multiple-ggplot2-plots-in-same.html) |
vp.layout<-function(x, y) viewport(layout.pos.row=x, layout.pos.col=y) |
arrange_ggplot2<-function(..., nrow=NULL, ncol=NULL, as.table=FALSE) { |
dots<-list(...) |
n<- length(dots) |
if(is.null(nrow) & is.null(ncol)) { nrow= floor(n/2) ; ncol= ceiling(n/nrow)} |
if(is.null(nrow)) { nrow= ceiling(n/ncol)} |
if(is.null(ncol)) { ncol= ceiling(n/nrow)} |
## NOTE see n2mfrow in grDevices for possible alternative |
grid.newpage() |
pushViewport(viewport(layout=grid.layout(nrow,ncol) ) ) |
ii.p<-1 |
for(ii.rowin seq(1, nrow)){ |
ii.table.row<-ii.row |
if(as.table) {ii.table.row<-nrow-ii.table.row+1} |
for(ii.colin seq(1, ncol)){ |
ii.table<-ii.p |
if(ii.p>n) break |
print(dots[[ii.table]], vp=vp.layout(ii.table.row, ii.col)) |
ii.p<-ii.p+1 |
} |
} |
} |
# Calculate DR payment (NOTE: Electricity savings potential ests are broken somehow...too high in some random cases...) |
dr.payment<-function(LMP,nbt.threshold,potential,load.shape,retail.elec,only.during.nbt=FALSE){ |
# LMP is a vector of LMP for the time period |
# nbt.threshold is a vector of nbt threshold times for the period (or a single value) |
# mw.potential is the maximum savings potential for the measure (a single value) |
# load shape is a vector of relative availability for the measure--fractions of peak (in time) |
# retail.elec is either a vector of prices or a single value |
timesteps<- length(LMP) |
if(length(nbt.threshold1)){nbt.threshold<-matrix(nbt.threshold,nrow=timesteps)} |
if(length(retail.elec1)){retail.elec<-matrix(retail.elec,nrow=timesteps)} |
if(length(load.shape)!=timesteps){stop('load shape different length than lmp')} |
potential<-potential*load.shape |
# make dr payment matrix with zeroes if < nbt and lMP if > nbt. |
dr.payment<-matrix(data=0,nrow=timesteps) |
pass.nbt<- which(LMP>=nbt.threshold) |
fail.nbt<- which(LMP<nbt.threshold) |
dr.payment[pass.nbt]<-LMP[pass.nbt] |
if(only.during.nbt){retail.elec[fail.nbt]<-0} |
dr.rev<-dr.payment*potential |
e.save<-retail.elec*potential |
tot<-dr.rev+e.save |
out<-data.frame(dr.rev=dr.rev,e.save=e.save,tot=tot) |
} #end function dr.payment |
Copy lines Copy permalink
Join GitHub today
Weather Data Csv Download
GitHub is home to over 36 million developers working together to host and review code, manage projects, and build software together.
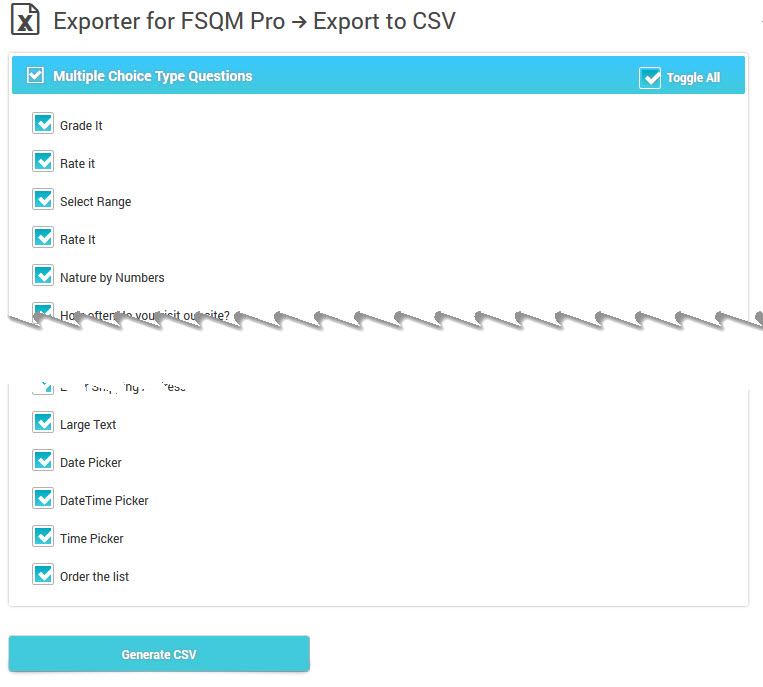
California Iso Download Csv Data Sheet
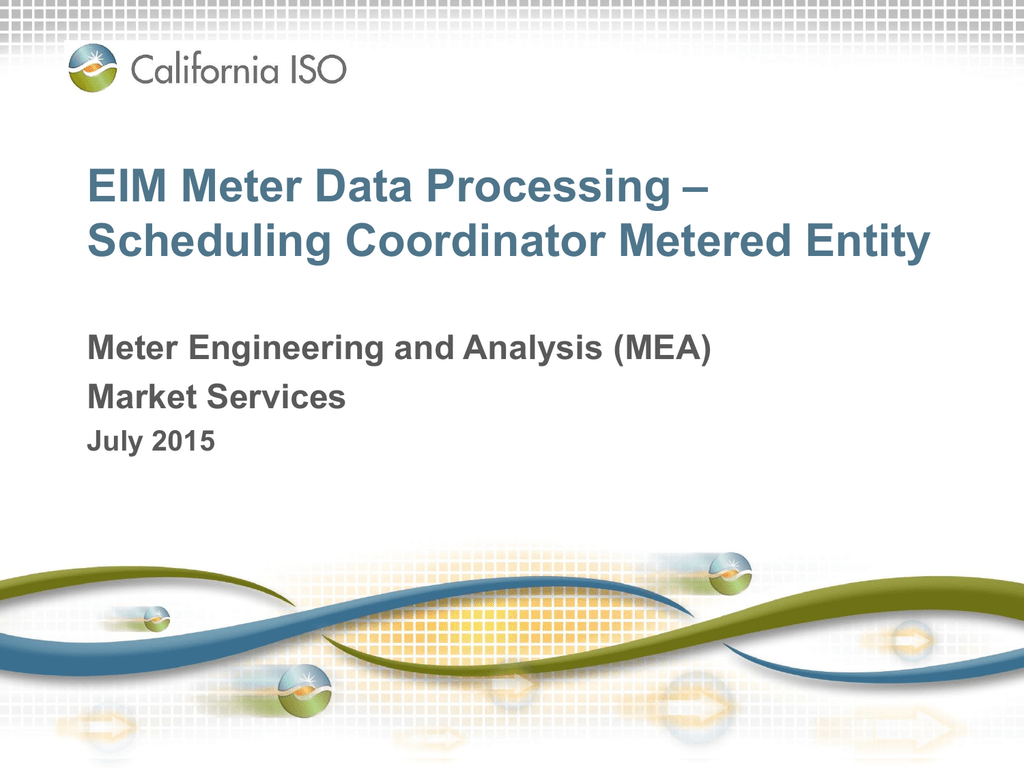
1 contributor
California Iso Download Csv Database
city | latd | longd | elevation_m | elevation_ft | population_total | area_total_sq_mi | area_land_sq_mi | area_water_sq_mi | area_total_km2 | area_land_km2 | area_water_km2 | area_water_percent | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | Adelanto | 34.57611111111112 | -117.43277777777779 | 875.0 | 2871.0 | 31765 | 56.027 | 56.00899999999999 | 0.018000000000000002 | 145.107 | 145.062 | 0.046 | 0.03 |
1 | AgouraHills | 34.15333333333333 | -118.76166666666667 | 281.0 | 922.0 | 20330 | 7.822 | 7.792999999999999 | 0.028999999999999998 | 20.26 | 20.184 | 0.076 | 0.37 |
2 | Alameda | 37.75611111111111 | -122.27444444444444 | 33.0 | 75467 | 22.96 | 10.610999999999999 | 12.349 | 59.465 | 27.482 | 31.983 | 53.79 | |
3 | Albany | 37.886944444444445 | -122.29777777777778 | 43.0 | 18969 | 5.465 | 1.788 | 3.677 | 14.155 | 4.632 | 9.524 | 67.28 | |
4 | Alhambra | 34.081944444444446 | -118.135 | 150.0 | 492.0 | 83089 | 7.632000000000001 | 7.631 | 0.001 | 19.766 | 19.762999999999998 | 0.003 | 0.01 |
5 | AlisoViejo | 33.575 | -117.72555555555556 | 127.0 | 417.0 | 47823 | 7.472 | 7.472 | 0.0 | 19.352 | 19.352 | 0.0 | 0.0 |
6 | Alturas | 41.48722222222222 | -120.5425 | 1332.0 | 4370.0 | 2827 | 2.449 | 2.435 | 0.013999999999999999 | 6.3420000000000005 | 6.306 | 0.036000000000000004 | 0.57 |
7 | AmadorCity | 38.419444444444444 | -120.82416666666666 | 280.0 | 919.0 | 185 | 0.314 | 0.314 | 0.0 | 0.813 | 0.813 | 0.0 | 0.0 |
8 | AmericanCanyon | 38.168055555555554 | -122.2525 | 14.0 | 46.0 | 19454 | 4.845 | 4.837 | 0.008 | 12.548 | 12.527000000000001 | 0.021 | 0.17 |
9 | Anaheim | 33.836111111111116 | -117.88972222222223 | 48.0 | 157.0 | 336000 | 50.81100000000001 | 49.835 | 0.976 | 131.6 | 129.07299999999998 | 2.5269999999999997 | 1.92 |
10 | Anderson | 40.452222222222225 | -122.29666666666667 | 132.0 | 430.0 | 9932 | 6.62 | 6.372000000000001 | 0.248 | 17.145 | 16.504 | 0.642 | 3.74 |
11 | AngelsCamp | 38.068333333333335 | -120.53972222222222 | 420.0 | 1378.0 | 3836 | 3.637 | 3.628 | 0.009000000000000001 | 9.421 | 9.397 | 0.024 | 0.25 |
12 | Antioch | 38.005 | -121.80583333333333 | 13.0 | 43.0 | 107100 | 29.083000000000002 | 28.349 | 0.7340000000000001 | 75.324 | 73.422 | 1.902 | 2.52 |
13 | AppleValley | 34.516666666666666 | -117.21666666666667 | 898.0 | 2946.0 | 69135 | 73.523 | 73.193 | 0.33 | 190.426 | 189.57 | 0.856 | 0.45 |
14 | Arcadia | 34.132777777777775 | -118.0363888888889 | 147.0 | 482.0 | 56364 | 11.133 | 10.925 | 0.20800000000000002 | 28.836 | 28.296 | 0.54 | 1.87 |
15 | Arcata | 40.86638888888889 | -124.08277777777778 | 23.0 | 17231 | 10.994000000000002 | 9.097000000000001 | 1.8969999999999998 | 28.473000000000003 | 23.561 | 4.912 | 17.25 | |
16 | ArroyoGrande | 35.12083333333334 | -120.58666666666666 | 36.0 | 118.0 | 17716 | 5.835 | 5.835 | 0.0 | 15.113 | 15.113 | 0.0 | 0.0 |
17 | Artesia | 33.867222222222225 | -118.08055555555555 | 16.0 | 52.0 | 16522 | 1.621 | 1.621 | 0.0 | 4.197 | 4.197 | 0.0 | 0.0 |
18 | Arvin | 35.20916666666667 | -118.82833333333333 | 137.0 | 449.0 | 19304 | 4.819 | 4.819 | 0.0 | 12.482000000000001 | 12.482000000000001 | 0.0 | 0.0 |
19 | Atascadero | 35.48416666666667 | -120.6725 | 268.0 | 879.0 | 28310 | 26.13 | 25.641 | 0.489 | 67.675 | 66.40899999999999 | 1.265 | 1.87 |
20 | Atherton | 37.45861111111111 | -122.2 | 18.0 | 59.0 | 6914 | 5.0489999999999995 | 5.0169999999999995 | 0.032 | 13.075999999999999 | 12.993 | 0.08199999999999999 | 0.63 |
21 | Atwater | 37.34777777777778 | -120.60916666666667 | 46.0 | 151.0 | 28168 | 6.096 | 6.087000000000001 | 0.009000000000000001 | 15.788 | 15.765999999999998 | 0.022000000000000002 | 0.14 |
22 | Auburn | 38.89861111111111 | -121.07444444444444 | 374.0 | 1227.0 | 13330 | 7.166 | 7.138 | 0.027999999999999997 | 18.56 | 18.488 | 0.071 | 0.38 |
23 | Avalon | 33.340833333333336 | -118.32777777777777 | 9.0 | 30.0 | 3728 | 2.937 | 2.935 | 0.002 | 7.607 | 7.602 | 0.005 | 0.07 |
24 | Avenal | 36.00416666666667 | -120.12888888888888 | 246.0 | 807.0 | 13239 | 19.422 | 19.422 | 0.0 | 50.302 | 50.302 | 0.0 | 0.0 |
25 | Azusa | 34.13055555555555 | -117.90694444444445 | 186.0 | 610.0 | 46361 | 9.669 | 9.656 | 0.013000000000000001 | 25.041999999999998 | 25.01 | 0.032 | 0.13 |
26 | Bakersfield | 35.36666666666667 | -119.01666666666667 | 404.0 | 347483 | 143.609 | 142.164 | 1.445 | 371.94599999999997 | 368.204 | 3.742 | 1.01 | |
27 | BaldwinPark | 34.08277777777778 | -117.97166666666666 | 114.0 | 374.0 | 75390 | 6.7860000000000005 | 6.631 | 0.155 | 17.575 | 17.174 | 0.4 | 2.28 |
28 | Banning | 33.931666666666665 | -116.89750000000001 | 716.0 | 2349.0 | 29603 | 23.099 | 23.099 | 0.0 | 59.826 | 59.826 | 0.0 | 0.0 |
29 | Barstow | 34.9 | -117.01666666666667 | 664.0 | 2178.0 | 22639 | 41.394 | 41.385 | 0.009000000000000001 | 107.209 | 107.186 | 0.023 | 0.02 |
30 | Beaumont | 33.924166666666665 | -116.97361111111111 | 796.0 | 2612.0 | 36877 | 30.926 | 30.912 | 0.013999999999999999 | 80.098 | 80.062 | 0.036000000000000004 | 0.04 |
31 | Bell | 33.983333333333334 | -118.18333333333334 | 43.0 | 141.0 | 35477 | 2.62 | 2.501 | 0.11900000000000001 | 6.7829999999999995 | 6.476 | 0.307 | 4.53 |
32 | Bellflower | 33.88805555555555 | -118.1275 | 22.0 | 71.0 | 76616 | 6.17 | 6.117000000000001 | 0.053 | 15.981 | 15.843 | 0.138 | 0.86 |
33 | BellGardens | 33.96805555555556 | -118.15611111111112 | 37.0 | 121.0 | 42072 | 2.463 | 2.459 | 0.004 | 6.379 | 6.367999999999999 | 0.012 | 0.18 |
34 | Belmont | 37.518055555555556 | -122.29166666666667 | 13.0 | 43.0 | 25835 | 4.63 | 4.621 | 0.009000000000000001 | 11.992 | 11.97 | 0.022000000000000002 | 0.19 |
35 | Belvedere | 37.87277777777778 | -122.46444444444445 | 11.0 | 36.0 | 2068 | 2.406 | 0.519 | 1.8869999999999998 | 6.234 | 1.345 | 4.888999999999999 | 78.42 |
36 | Benicia | 38.06333333333333 | -122.15611111111112 | 8.0 | 26.0 | 26997 | 15.72 | 12.929 | 2.7910000000000004 | 40.714 | 33.486 | 7.229 | 17.75 |
37 | Berkeley | 37.87166666666667 | -122.27277777777778 | 200.0 | 660.0 | 112580 | 17.695999999999998 | 10.47 | 7.226 | 45.833 | 27.118000000000002 | 18.715999999999998 | 40.83 |
38 | BeverlyHills | 34.073055555555555 | -118.39944444444446 | 79.0 | 259.0 | 34290 | 5.71 | 5.707999999999999 | 0.002 | 14.79 | 14.784 | 0.006 | 0.04 |
39 | BigBearLake | 34.24138888888889 | -116.90333333333334 | 2058.0 | 6752.0 | 5019 | 6.534 | 6.346 | 0.188 | 16.923 | 16.435 | 0.488 | 2.88 |
40 | Biggs | 39.413888888888884 | -121.71027777777778 | 30.0 | 98.0 | 1707 | 0.636 | 0.636 | 0.0 | 1.646 | 1.646 | 0.0 | 0.0 |
41 | Bishop | 37.3635 | -118.3951 | 4150.0 | 3879 | 1.911 | 1.864 | 0.047 | 4.949 | 4.827 | 0.122 | 2.5 | |
42 | BlueLake | 40.882777777777775 | -123.98388888888888 | 40.0 | 131.0 | 1253 | 0.622 | 0.5920000000000001 | 0.03 | 1.61 | 1.5330000000000001 | 0.077 | 4.8 |
43 | Blythe | 33.61027777777778 | -114.59638888888888 | 83.0 | 272.0 | 20817 | 26.971999999999998 | 26.189 | 0.7829999999999999 | 69.855 | 67.828 | 2.0269999999999997 | 2.9 |
44 | Bradbury | 34.14944444444444 | -117.97444444444444 | 206.0 | 676.0 | 1048 | 1.9580000000000002 | 1.9569999999999999 | 0.001 | 5.073 | 5.07 | 0.003 | 0.06 |
45 | Brawley | 32.978611111111114 | -115.53027777777778 | -34.0 | -112.0 | 24953 | 7.682 | 7.682 | 0.0 | 19.895 | 19.895 | 0.0 | 0.0 |
46 | Brea | 33.92333333333333 | -117.8888888888889 | 110.0 | 361.0 | 39282 | 12.109000000000002 | 12.078 | 0.031 | 31.363000000000003 | 31.283 | 0.08 | 0.26 |
47 | Brentwood | 37.93194444444444 | -121.69583333333334 | 79.0 | 51481 | 14.805 | 14.786 | 0.019 | 38.345 | 38.295 | 0.049 | 0.13 | |
48 | Brisbane | 37.68083333333333 | -122.41916666666667 | 33.0 | 108.0 | 4282 | 20.077 | 3.096 | 16.980999999999998 | 51.998999999999995 | 8.017000000000001 | 43.981 | 84.58 |
49 | Buellton | 34.61416666666667 | -120.19388888888889 | 109.0 | 358.0 | 4828 | 1.5830000000000002 | 1.5819999999999999 | 0.001 | 4.099 | 4.098 | 0.002 | 0.04 |
50 | BuenaPark | 33.85611111111111 | -118.00416666666666 | 23.0 | 75.0 | 80530 | 10.552999999999999 | 10.524000000000001 | 0.028999999999999998 | 27.331999999999997 | 27.256999999999998 | 0.075 | 0.28 |
51 | Burbank | 34.180277777777775 | -118.32833333333333 | 185.0 | 607.0 | 103340 | 17.379 | 17.340999999999998 | 0.038 | 45.011 | 44.913000000000004 | 0.098 | 0.22 |
52 | Burlingame | 37.583333333333336 | -122.36361111111111 | 12.0 | 39.0 | 28806 | 6.057 | 4.406000000000001 | 1.651 | 15.686 | 11.411 | 4.275 | 27.25 |
53 | Calabasas | 34.138333333333335 | -118.66083333333334 | 243.0 | 5.0 | 23058 | 13.3 | 13.249 | 0.051 | 34.4 | 34.27 | 0.131 | 0.38 |
54 | Calexico | 32.678888888888885 | -115.49888888888889 | 3.0 | 38572 | 8.391 | 8.391 | 0.0 | 21.733 | 21.733 | 0.0 | 0.0 | |
55 | CaliforniaCity | 35.12583333333333 | -117.98583333333333 | 733.0 | 2405.0 | 14120 | 203.63099999999997 | 203.523 | 0.10800000000000001 | 527.401 | 527.122 | 0.27899999999999997 | 0.05 |
56 | Calimesa | 33.988055555555555 | -117.04305555555555 | 729.0 | 2392.0 | 7879 | 14.847000000000001 | 14.847000000000001 | 0.0 | 38.454 | 38.454 | 0.0 | 0.0 |
57 | Calipatria | 33.12555555555556 | -115.51416666666667 | -180.0 | 7705 | 3.716 | 3.716 | 0.0 | 9.624 | 9.624 | 0.0 | 0.0 | |
58 | Calistoga | 38.581388888888895 | -122.58277777777778 | 106.0 | 348.0 | 5155 | 2.613 | 2.595 | 0.018000000000000002 | 6.769 | 6.722 | 0.047 | 0.7 |
59 | Camarillo | 34.233333333333334 | -119.03333333333333 | 54.0 | 177.0 | 65201 | 19.543 | 19.528 | 0.015 | 50.617 | 50.577 | 0.04 | 0.08 |
60 | Campbell | 37.28388888888889 | -121.955 | 60.0 | 197.0 | 39349 | 5.886 | 5.797999999999999 | 0.08800000000000001 | 15.245 | 15.017000000000001 | 0.228 | 1.49 |
61 | CanyonLake | 33.68416666666666 | -117.25555555555556 | 422.0 | 1385.0 | 10561 | 4.671 | 3.928 | 0.743 | 12.099 | 10.173 | 1.926 | 15.92 |
62 | Capitola | 36.97638888888889 | -121.95472222222223 | 4.0 | 13.0 | 9918 | 1.676 | 1.5930000000000002 | 0.083 | 4.34 | 4.126 | 0.214 | 4.92 |
63 | Carlsbad | 33.121944444444445 | -117.29694444444445 | 16.0 | 52.0 | 105328 | 39.11 | 37.722 | 1.3880000000000001 | 101.295 | 97.699 | 3.596 | 3.55 |
64 | CarmelbytheSea | 36.555277777777775 | -121.92333333333333 | 3722 | 1.08 | 1.08 | 0.0 | 2.798 | 2.798 | 0.0 | 0.0 | ||
65 | Carpinteria | 34.399166666666666 | -119.51638888888888 | 10.0 | 33.0 | 13040 | 9.272 | 2.5860000000000003 | 6.686 | 24.011999999999997 | 6.697 | 17.315 | 72.11 |
66 | Carson | 33.83972222222222 | -118.25972222222222 | 8.2 | 27.0 | 91714 | 18.968 | 18.724 | 0.244 | 49.126999999999995 | 48.495 | 0.631 | 1.29 |
67 | CathedralCity | 33.80777777777777 | -116.46472222222222 | 100.0 | 328.0 | 51200 | 21.756 | 21.499000000000002 | 0.257 | 56.349 | 55.683 | 0.6659999999999999 | 1.18 |
68 | Ceres | 37.60138888888889 | -120.95722222222223 | 28.0 | 92.0 | 45417 | 8.019 | 8.011000000000001 | 0.008 | 20.771 | 20.749000000000002 | 0.022000000000000002 | 0.1 |
69 | Cerritos | 33.86833333333333 | -118.0675 | 14.0 | 34.0 | 49041 | 8.856 | 8.725 | 0.131 | 22.936999999999998 | 22.598000000000003 | 0.33899999999999997 | 1.48 |
70 | Chico | 39.74 | -121.83555555555554 | 74.0 | 245.0 | 86187 | 33.095 | 32.923 | 0.172 | 85.71600000000001 | 85.271 | 0.446 | 0.52 |
71 | Chino | 34.01777777777777 | -117.69 | 222.0 | 728.0 | 77983 | 29.651999999999997 | 29.639 | 0.013000000000000001 | 76.79899999999999 | 76.766 | 0.033 | 0.04 |
72 | ChinoHills | 33.97527777777778 | -117.72305555555556 | 365.0 | 1070.0 | 75655 | 44.748999999999995 | 44.681000000000004 | 0.068 | 115.899 | 115.723 | 0.175 | 0.15 |
73 | Chowchilla | 37.11666666666667 | -120.26666666666667 | 73.0 | 240.0 | 18720 | 7.6610000000000005 | 7.6610000000000005 | 0.0 | 19.842 | 19.842 | 0.0 | 0.0 |
74 | ChulaVista | 32.62777777777778 | -117.04805555555555 | 21.0 | 69.0 | 243916 | 52.093999999999994 | 49.631 | 2.463 | 134.925 | 128.545 | 6.38 | 4.73 |
75 | CitrusHeights | 38.7 | -121.28333333333333 | 50.0 | 164.0 | 83301 | 14.228 | 14.228 | 0.0 | 36.851 | 36.851 | 0.0 | 0.0 |
76 | Claremont | 34.11 | -117.71972222222223 | 34926 | 13.485999999999999 | 13.347999999999999 | 0.138 | 34.93 | 34.571 | 0.358 | 1.03 | ||
77 | Clayton | 37.941111111111105 | -121.93583333333333 | 120.0 | 394.0 | 10897 | 3.8360000000000003 | 3.8360000000000003 | 0.0 | 9.935 | 9.935 | 0.0 | 0.0 |
78 | Clearlake | 38.958333333333336 | -122.62638888888888 | 432.0 | 1417.0 | 15250 | 10.581 | 10.129 | 0.452 | 27.404 | 26.234 | 1.17 | 4.27 |
79 | Cloverdale | 38.799166666666665 | -123.01722222222222 | 335.0 | 8618 | 2.648 | 2.648 | 0.0 | 6.857 | 6.857 | 0.0 | 0.0 | |
80 | Clovis | 36.82527777777778 | -119.70305555555557 | 110.0 | 361.0 | 101314 | 23.278000000000002 | 23.278000000000002 | 0.0 | 60.288999999999994 | 60.288999999999994 | 0.0 | 0.0 |
81 | Coachella | 33.67944444444444 | -116.17444444444445 | -20.74 | -66.0 | 40704 | 28.95 | 28.95 | 0.0 | 74.98100000000001 | 74.98100000000001 | 0.0 | 0.0 |
82 | Coalinga | 36.13972222222222 | -120.36027777777777 | 205.0 | 673.0 | 13380 | 6.15 | 6.119 | 0.031 | 15.927 | 15.847000000000001 | 0.08 | 0.5 |
83 | Colfax | 39.09722222222222 | -120.9538888888889 | 739.0 | 2425.0 | 1963 | 1.4069999999999998 | 1.4069999999999998 | 0.0 | 3.645 | 3.645 | 0.0 | 0.0 |
84 | Colma | 37.678888888888885 | -122.45555555555556 | 37.0 | 121.0 | 1792 | 1.909 | 1.909 | 0.0 | 4.945 | 4.945 | 0.0 | 0.0 |
85 | Colton | 34.065 | -117.32166666666666 | 306.0 | 1004.0 | 52154 | 16.039 | 15.324000000000002 | 0.715 | 41.541000000000004 | 39.689 | 1.8519999999999999 | 4.46 |
86 | Colusa | 39.214444444444446 | -122.00944444444444 | 16.0 | 52.0 | 5971 | 1.834 | 1.834 | 0.0 | 4.751 | 4.751 | 0.0 | 0.0 |
87 | Commerce | 34.00055555555556 | -118.15472222222223 | 43.0 | 141.0 | 12823 | 6.537999999999999 | 6.537000000000001 | 0.001 | 16.933 | 16.93 | 0.003 | 0.02 |
88 | Compton | 33.89666666666667 | -118.22500000000001 | 21.0 | 69.0 | 96455 | 10.116 | 10.012 | 0.10400000000000001 | 26.201999999999998 | 25.932 | 0.27 | 1.03 |
89 | Concord | 37.97805555555556 | -122.03111111111112 | 26.0 | 85.3 | 122067 | 30.546 | 30.546 | 0.0 | 79.11399999999999 | 79.11399999999999 | 0.0 | 0.0 |
90 | Corcoran | 36.09805555555556 | -119.56027777777777 | 63.0 | 207.0 | 25515 | 7.4670000000000005 | 7.4670000000000005 | 0.0 | 19.338 | 19.338 | 0.0 | 0.0 |
91 | Corning | 39.92611111111111 | -122.18055555555556 | 84.0 | 276.0 | 17382 | 3.55 | 3.55 | 0.0 | 9.193 | 9.193 | 0.0 | 0.0 |
92 | Corona | 33.86666666666667 | -117.56666666666666 | 206.0 | 678.0 | 158391 | 38.93 | 38.825 | 0.105 | 100.829 | 100.55799999999999 | 0.272 | 0.27 |
93 | Coronado | 32.67805555555555 | -117.1725 | 6.0 | 20.0 | 24697 | 32.666 | 7.931 | 24.735 | 84.603 | 20.541 | 64.062 | 75.72 |
94 | CorteMadera | 37.925555555555555 | -122.5275 | 12.0 | 39.0 | 9253 | 4.406000000000001 | 3.1639999999999997 | 1.242 | 11.41 | 8.193 | 3.216 | 28.19 |
95 | CostaMesa | 33.665 | -117.91222222222223 | 30.0 | 98.0 | 109960 | 15.7 | 15.654000000000002 | 0.046 | 40.662 | 40.543 | 0.11900000000000001 | 0.29 |
96 | Cotati | 38.32777777777778 | -122.70916666666668 | 34.0 | 112.0 | 7310 | 1.883 | 1.88 | 0.003 | 4.877 | 4.869 | 0.008 | 0.17 |
97 | Covina | 34.09166666666667 | -117.87916666666666 | 170.0 | 558.0 | 47796 | 7.041 | 7.026 | 0.015 | 18.236 | 18.195999999999998 | 0.039 | 0.22 |
98 | CrescentCity | 41.755833333333335 | -124.20166666666667 | 43.0 | 7643 | 2.415 | 1.963 | 0.452 | 6.255 | 5.085 | 1.17 | 18.7 | |
99 | Cudahy | 33.96416666666667 | -118.1825 | 37.0 | 121.0 | 23805 | 1.226 | 1.175 | 0.051 | 3.175 | 3.043 | 0.132 | 4.15 |
100 | CulverCity | 34.007777777777775 | -118.40083333333334 | 29.0 | 95.0 | 38883 | 5.138999999999999 | 5.111000000000001 | 0.027999999999999997 | 13.31 | 13.238 | 0.07200000000000001 | 0.54 |
101 | Cupertino | 37.3175 | -122.04194444444444 | 72.0 | 236.0 | 58302 | 11.257 | 11.255999999999998 | 0.001 | 29.156 | 29.153000000000002 | 0.003 | 0.01 |
102 | Cypress | 33.818333333333335 | -118.03916666666666 | 12.0 | 39.0 | 47802 | 6.59 | 6.581 | 0.009000000000000001 | 17.069000000000003 | 17.045 | 0.024 | 0.14 |
103 | DalyCity | 37.686388888888885 | -122.46833333333333 | 57.0 | 187.0 | 101123 | 7.664 | 7.664 | 0.0 | 19.849 | 19.849 | 0.0 | 0.0 |
104 | DanaPoint | 33.467222222222226 | -117.69805555555556 | 44.0 | 144.0 | 33351 | 29.484 | 6.497000000000001 | 22.987 | 76.36399999999999 | 16.828 | 59.536 | 77.96 |
105 | Danville | 37.82166666666667 | -122.0 | 109.0 | 358.0 | 42039 | 18.028 | 18.028 | 0.0 | 46.693000000000005 | 46.693000000000005 | 0.0 | 0.0 |
106 | Davis | 38.553888888888885 | -121.73805555555556 | 16.0 | 52.0 | 65622 | 9.919 | 9.887 | 0.032 | 25.69 | 25.608 | 0.08199999999999999 | 0.32 |
107 | Delano | 35.76888888888889 | -119.24694444444445 | 96.0 | 315.0 | 53819 | 14.355 | 14.302999999999999 | 0.052000000000000005 | 37.18 | 37.044000000000004 | 0.135 | 0.36 |
108 | DelMar | 32.955000000000005 | -117.26388888888889 | 34.0 | 112.0 | 4161 | 1.777 | 1.7069999999999999 | 0.07 | 4.602 | 4.421 | 0.18100000000000002 | 3.94 |
109 | DelReyOaks | 36.593333333333334 | -121.835 | 25.0 | 82.0 | 1624 | 0.483 | 0.48100000000000004 | 0.002 | 1.251 | 1.246 | 0.005 | 0.42 |
110 | DesertHotSprings | 33.961111111111116 | -116.50805555555556 | 328.0 | 1076.0 | 25938 | 23.642 | 23.615 | 0.027000000000000003 | 61.233000000000004 | 61.163999999999994 | 0.069 | 0.11 |
111 | DiamondBar | 34.001666666666665 | -117.82083333333333 | 212.0 | 696.0 | 55544 | 14.885 | 14.88 | 0.005 | 38.552 | 38.538000000000004 | 0.013999999999999999 | 0.04 |
112 | Dinuba | 36.544999999999995 | -119.38916666666667 | 102.0 | 345.0 | 21453 | 6.47 | 6.47 | 0.0 | 16.758 | 16.758 | 0.0 | 0.0 |
113 | Dixon | 38.44916666666666 | -121.82694444444444 | 19.0 | 62.0 | 18351 | 7.0920000000000005 | 6.996 | 0.096 | 18.368 | 18.118 | 0.249 | 1.36 |
114 | Dorris | 41.965 | -121.91888888888889 | 1294.0 | 4245.0 | 939 | 0.718 | 0.7020000000000001 | 0.016 | 1.86 | 1.819 | 0.040999999999999995 | 2.19 |
115 | DosPalos | 36.983333333333334 | -120.63333333333334 | 362.0 | 118.0 | 4950 | 1.35 | 1.35 | 0.0 | 3.4960000000000004 | 3.4960000000000004 | 0.0 | 0.0 |
116 | Downey | 33.93805555555555 | -118.13083333333333 | 36.0 | 118.0 | 111772 | 12.568 | 12.408 | 0.16 | 32.551 | 32.137 | 0.414 | 1.27 |
117 | Duarte | 34.140277777777776 | -117.96166666666667 | 156.0 | 512.0 | 21321 | 6.69 | 6.69 | 0.0 | 17.328 | 17.328 | 0.0 | 0.0 |
118 | Dublin | 37.702222222222225 | -121.93583333333333 | 367.0 | 49890 | 14.912 | 14.908 | 0.004 | 38.622 | 38.611 | 0.011000000000000001 | 0.03 | |
119 | Dunsmuir | 41.22166666666667 | -122.27305555555556 | 695.0 | 2280.0 | 1650 | 1.735 | 1.6980000000000002 | 0.037000000000000005 | 4.494 | 4.398 | 0.096 | 2.14 |
120 | EastPaloAlto | 37.466944444444444 | -122.13972222222223 | 6.0 | 20.0 | 28155 | 2.612 | 2.505 | 0.107 | 6.766 | 6.4879999999999995 | 0.278 | 4.11 |
121 | Eastvale | 33.963055555555556 | -117.56388888888888 | 627.0 | 53668 | 11.445 | 11.405 | 0.04 | 29.644000000000002 | 29.539 | 0.10400000000000001 | 0.35 | |
122 | ElCajon | 32.79833333333333 | -116.96000000000001 | 133.0 | 436.0 | 99478 | 14.433 | 14.433 | 0.0 | 37.381 | 37.381 | 0.0 | 0.0 |
123 | ElCentro | 32.8 | -115.56666666666666 | -12.0 | -39.0 | 42598 | 11.099 | 11.081 | 0.018000000000000002 | 28.746 | 28.7 | 0.046 | 0.16 |
124 | ElCerrito | 37.91583333333333 | -122.31166666666667 | 69.0 | 23549 | 3.688 | 3.688 | 0.0 | 9.551 | 9.551 | 0.0 | 0.0 | |
125 | ElkGrove | 38.43833333333333 | -121.38194444444444 | 14.0 | 45.0 | 153015 | 42.239 | 42.19 | 0.049 | 109.398 | 109.271 | 0.127 | 0.12 |
126 | ElMonte | 34.07333333333334 | -118.0275 | 91.0 | 299.0 | 113475 | 9.648 | 9.562000000000001 | 0.086 | 24.988000000000003 | 24.766 | 0.222 | 0.89 |
127 | ElSegundo | 33.921388888888885 | -118.40611111111112 | 35.0 | 115.0 | 16654 | 5.465 | 5.462999999999999 | 0.002 | 14.152000000000001 | 14.148 | 0.004 | 0.03 |
128 | Emeryville | 37.831388888888895 | -122.28527777777778 | 7.0 | 23.0 | 10080 | 2.01 | 1.246 | 0.764 | 5.206 | 3.2260000000000004 | 1.979 | 38.02 |
129 | Encinitas | 33.044444444444444 | -117.27166666666666 | 25.0 | 82.0 | 59518 | 19.99 | 18.812 | 1.178 | 51.772 | 48.722 | 3.05 | 5.89 |
130 | Escalon | 37.791666666666664 | -120.99166666666666 | 36.0 | 118.0 | 7266 | 2.3680000000000003 | 2.301 | 0.067 | 6.1339999999999995 | 5.959 | 0.175 | 2.85 |
131 | Escondido | 33.124722222222225 | -117.08083333333333 | 197.0 | 646.0 | 143911 | 36.989000000000004 | 36.813 | 0.17600000000000002 | 95.801 | 95.345 | 0.456 | 0.48 |
132 | Etna | 41.45722222222223 | -122.89694444444446 | 895.0 | 2936.0 | 737 | 0.759 | 0.758 | 0.001 | 1.965 | 1.962 | 0.002 | 0.12 |
133 | Eureka | 40.801944444444445 | -124.16361111111112 | 39.0 | 27191 | 14.454 | 9.384 | 5.07 | 37.435 | 24.305 | 13.13 | 35.07 | |
134 | Exeter | 36.29416666666666 | -119.14277777777778 | 119.0 | 390.0 | 10334 | 2.463 | 2.463 | 0.0 | 6.379 | 6.379 | 0.0 | 0.0 |
135 | Fairfax | 37.98722222222222 | -122.58888888888889 | 35.0 | 115.0 | 7441 | 2.204 | 2.204 | 0.0 | 5.707000000000001 | 5.707000000000001 | 0.0 | 0.0 |
136 | Fairfield | 38.257777777777775 | -122.05416666666666 | 4.0 | 13.0 | 108321 | 37.635 | 34.39 | 3.245 | 97.475 | 94.839 | 2.635 | 5.65 |
137 | Farmersville | 36.301111111111105 | -119.2075 | 109.0 | 358.0 | 10588 | 2.258 | 2.258 | 0.0 | 5.849 | 5.849 | 0.0 | 0.0 |
138 | Ferndale | 40.57666666666667 | -124.26333333333334 | 17.0 | 56.0 | 1371 | 1.0270000000000001 | 1.0270000000000001 | 0.0 | 2.659 | 2.659 | 0.0 | 0.0 |
139 | Fillmore | 34.40138888888889 | -118.91777777777779 | 139.0 | 456.0 | 15002 | 3.365 | 3.364 | 0.001 | 8.715 | 8.712 | 0.003 | 0.03 |
140 | Firebaugh | 36.85888888888889 | -120.45611111111111 | 46.0 | 151.0 | 7549 | 3.5189999999999997 | 3.4619999999999997 | 0.057 | 9.113999999999999 | 8.967 | 0.14800000000000002 | 1.62 |
141 | Folsom | 38.67222222222222 | -121.15777777777778 | 67.0 | 220.0 | 72203 | 24.301 | 21.945 | 2.356 | 62.93899999999999 | 56.838 | 6.101 | 9.69 |
142 | Fontana | 34.1 | -117.46666666666667 | 377.0 | 1237.0 | 201812 | 42.431999999999995 | 42.431999999999995 | 3.0 | 109.899 | 109.899 | 6.0 | 3.0 |
143 | FortBragg | 39.44583333333333 | -123.80527777777777 | 26.0 | 85.0 | 7273 | 2.8 | 2.7 | 7.2 | 7.1 | 0.1 | ||
144 | FortJones | 41.60722222222223 | -122.84194444444444 | 842.0 | 2762.0 | 839 | 0.602 | 0.602 | 0.0 | 1.56 | 1.56 | 0.0 | 0.0 |
145 | Fortuna | 40.59805555555556 | -124.15722222222223 | 20.0 | 66.0 | 11926 | 4.845 | 4.845 | 0.0 | 12.549000000000001 | 12.549000000000001 | 0.0 | 0.0 |
146 | FosterCity | 37.55138888888889 | -122.26638888888888 | 2.0 | 7.0 | 30567 | 19.840999999999998 | 3.7560000000000002 | 16.085 | 51.388000000000005 | 9.729 | 41.659 | 81.07 |
147 | FountainValley | 33.70861111111111 | -117.9563888888889 | 10.0 | 33.0 | 55313 | 9.031 | 9.017999999999999 | 0.013000000000000001 | 23.391 | 23.357 | 0.034 | 0.14 |
148 | Fowler | 36.63333333333333 | -119.68333333333334 | 94.0 | 308.0 | 5570 | 2.531 | 2.531 | 0.0 | 6.556 | 6.556 | 0.0 | 0.0 |
149 | Fremont | 37.54833333333333 | -121.98861111111111 | 71.0 | 218.0 | 220000 | 87.61 | 77.459 | 10.151 | 226.91 | 200.618 | 26.291999999999998 | 11.59 |
150 | Fresno | 36.75 | -119.76666666666667 | 308.0 | 509039 | 112.30799999999999 | 111.95700000000001 | 0.35100000000000003 | 290.877 | 289.967 | 0.91 | 0.31 | |
151 | Fullerton | 33.88 | -117.92861111111111 | 50.0 | 164.0 | 135161 | 22.36 | 22.353 | 0.011000000000000001 | 57.92100000000001 | 57.893 | 0.027000000000000003 | 0.05 |
152 | Galt | 38.26083333333333 | -121.30305555555556 | 16.0 | 47.0 | 23647 | 5.944 | 5.931 | 0.013000000000000001 | 15.397 | 15.362 | 0.035 | 0.23 |
153 | Gardena | 33.89361111111111 | -118.30777777777777 | 15.0 | 49.0 | 58829 | 5.865 | 5.829 | 0.036000000000000004 | 15.190999999999999 | 15.097000000000001 | 0.094 | 0.62 |
154 | GardenGrove | 33.778888888888886 | -117.96027777777778 | 27.0 | 89.0 | 170883 | 17.959 | 17.941 | 0.018000000000000002 | 46.513000000000005 | 46.467 | 0.046 | 0.1 |
155 | Gilroy | 37.011944444444445 | -121.58 | 61.0 | 200.0 | 48821 | 16.156 | 16.146 | 0.01 | 41.845 | 41.818999999999996 | 0.027000000000000003 | 0.06 |
156 | Glendale | 34.170833333333334 | -118.25 | 159.0 | 522.0 | 196021 | 30.583000000000002 | 30.453000000000003 | 0.13 | 79.212 | 78.874 | 0.33799999999999997 | 0.43 |
157 | Glendora | 34.130833333333335 | -117.85416666666666 | 236.0 | 774.0 | 50073 | 19.558 | 19.393 | 0.165 | 50.655 | 50.228 | 0.42700000000000005 | 0.84 |
158 | Goleta | 34.440555555555555 | -119.81361111111111 | 20.0 | 29888 | 7.975 | 7.903 | 0.07200000000000001 | 20.654 | 20.467 | 0.187 | 0.9 | |
159 | Gonzales | 36.50666666666667 | -121.44444444444444 | 41.0 | 135.0 | 8187 | 1.959 | 1.921 | 0.038 | 5.075 | 4.976 | 0.098 | 1.93 |
160 | GrandTerrace | 34.03111111111111 | -117.31666666666666 | 324.0 | 1063.0 | 12040 | 3.502 | 3.502 | 0.0 | 9.07 | 9.07 | 0.0 | 0.0 |
161 | GrassValley | 39.219166666666666 | -121.05833333333334 | 735.0 | 2411.0 | 12860 | 4.743 | 4.743 | 0.0 | 12.285 | 12.285 | 0.0 | 0.0 |
162 | Greenfield | 36.32083333333334 | -121.24388888888889 | 88.0 | 289.0 | 16330 | 2.135 | 2.135 | 0.0 | 5.53 | 5.53 | 0.0 | 0.0 |
163 | Gridley | 39.36388888888889 | -121.69361111111111 | 29.0 | 95.0 | 6593 | 2.07 | 0.0 | 2.07 | 0.0 | 0.0 | ||
164 | GroverBeach | 35.12083333333334 | -120.61944444444444 | 19.0 | 62.0 | 13156 | 2.314 | 2.31 | 0.004 | 5.995 | 5.983 | 0.011000000000000001 | 0.19 |
165 | Guadalupe | 34.96555555555556 | -120.57305555555556 | 26.0 | 85.0 | 7080 | 1.314 | 1.3090000000000002 | 0.005 | 3.405 | 3.391 | 0.013999999999999999 | 0.4 |
166 | Gustine | 37.257777777777775 | -120.99888888888889 | 30.0 | 98.0 | 5520 | 1.551 | 1.551 | 0.0 | 4.0169999999999995 | 4.0169999999999995 | 0.0 | 0.0 |
167 | HalfMoonBay | 37.45888888888889 | -122.43694444444445 | 22.0 | 72.0 | 11324 | 6.444 | 6.4239999999999995 | 0.02 | 16.692 | 16.639 | 0.053 | 0.32 |
168 | Hanford | 36.3275 | -119.64555555555556 | 76.0 | 249.0 | 55283 | 16.589000000000002 | 16.589000000000002 | 0.0 | 42.966 | 42.966 | 0.0 | 0.0 |
169 | HawaiianGardens | 33.828611111111115 | -118.0736111111111 | 10.0 | 33.0 | 14254 | 0.956 | 0.946 | 0.01 | 2.477 | 2.45 | 0.027000000000000003 | 1.09 |
170 | Hawthorne | 33.91722222222222 | -118.34861111111111 | 22.0 | 72.0 | 84293 | 6.0920000000000005 | 6.081 | 0.011000000000000001 | 15.779000000000002 | 15.75 | 0.028999999999999998 | 0.18 |
171 | Hayward | 37.66888888888889 | -122.08083333333333 | 105.0 | 149392 | 63.748000000000005 | 45.323 | 18.425 | 165.108 | 117.38600000000001 | 47.721000000000004 | 28.9 | |
172 | Healdsburg | 38.6175 | -122.86638888888888 | 32.0 | 105.0 | 11254 | 4.4639999999999995 | 4.457 | 0.006999999999999999 | 11.561 | 11.543 | 0.018000000000000002 | 0.15 |
173 | Hemet | 33.74194444444444 | -116.98305555555555 | 486.0 | 1594.0 | 78657 | 27.846999999999998 | 27.846999999999998 | 0.0 | 72.124 | 72.124 | 0.0 | 0.0 |
174 | Hercules | 38.01722222222222 | -122.28861111111111 | 24.0 | 79.0 | 24060 | 18.179000000000002 | 6.205 | 11.974 | 47.083999999999996 | 16.072 | 31.011999999999997 | 65.87 |
175 | HermosaBeach | 33.86638888888889 | -118.39972222222222 | 8.0 | 26.0 | 19506 | 1.4269999999999998 | 1.4269999999999998 | 0.0 | 3.695 | 3.695 | 0.0 | 0.0 |
176 | Hesperia | 34.41277777777778 | -117.30611111111111 | 971.0 | 3186.0 | 90173 | 73.209 | 73.096 | 0.113 | 189.61 | 189.31599999999997 | 0.294 | 0.15 |
177 | HiddenHills | 34.1675 | -118.66083333333334 | 328.0 | 1076.0 | 1856 | 1.689 | 1.689 | 0.0 | 4.373 | 4.373 | 0.0 | 0.0 |
178 | Highland | 34.11833333333333 | -117.2025 | 399.0 | 1309.0 | 53014 | 18.89 | 18.755 | 0.135 | 48.924 | 48.575 | 0.349 | 0.71 |
179 | Hillsborough | 37.56027777777778 | -122.35638888888889 | 98.0 | 322.0 | 10825 | 6.19 | 6.19 | 0.0 | 16.031 | 16.031 | 0.0 | 0.0 |
180 | Hollister | 36.84722222222222 | -121.39833333333334 | 88.0 | 289.0 | 34928 | 7.29 | 7.29 | 0.0 | 18.88 | 18.88 | 0.0 | 0.0 |
181 | Holtville | 32.81111111111111 | -115.38027777777778 | -3.0 | -10.0 | 5939 | 1.153 | 1.148 | 0.005 | 2.986 | 2.9739999999999998 | 0.012 | 0.4 |
182 | Hughson | 37.60305555555556 | -120.86694444444444 | 38.0 | 125.0 | 6640 | 1.815 | 1.815 | 0.0 | 4.7010000000000005 | 4.7010000000000005 | 0.0 | 0.0 |
183 | HuntingtonBeach | 33.69277777777778 | -118.00027777777778 | 12.0 | 39.0 | 197575 | 31.881999999999998 | 26.748 | 5.1339999999999995 | 82.57600000000001 | 69.278 | 13.298 | 16.1 |
184 | HuntingtonPark | 33.983333333333334 | -118.21666666666667 | 52.0 | 171.0 | 58114 | 3.016 | 3.013 | 0.003 | 7.811 | 7.8020000000000005 | 0.008 | 0.11 |
185 | Huron | 36.20277777777778 | -120.10305555555556 | 114.0 | 374.0 | 6754 | 1.591 | 1.591 | 0.0 | 4.121 | 4.121 | 0.0 | 0.0 |
186 | ImperialBeach | 32.57833333333333 | -117.11722222222221 | 21.0 | 69.0 | 26324 | 4.485 | 4.1610000000000005 | 0.324 | 11.616 | 10.777999999999999 | 0.838 | 7.22 |
187 | Imperial | 32.847500000000004 | -115.56944444444444 | -18.0 | -59.0 | 14758 | 5.856 | 5.856 | 0.0 | 15.168 | 15.168 | 0.0 | 0.0 |
188 | IndianWells | 33.715833333333336 | -116.3411111111111 | 27.0 | 89.0 | 4958 | 14.591 | 14.321 | 0.27 | 37.79 | 37.091 | 0.6990000000000001 | 1.85 |
189 | Indio | 33.72 | -116.23194444444445 | -4.0 | -13.0 | 76036 | 29.189 | 29.180999999999997 | 0.008 | 75.6 | 75.579 | 0.021 | 0.03 |
190 | Industry | 34.016666666666666 | -117.95 | 98.0 | 322.0 | 219 | 12.064 | 11.785 | 0.27899999999999997 | 31.245 | 30.522 | 0.723 | 2.31 |
191 | Inglewood | 33.9575 | -118.3461111111111 | 40.0 | 131.0 | 109673 | 9.093 | 9.068 | 0.025 | 23.549 | 23.486 | 0.064 | 0.27 |
192 | Ione | 38.35277777777778 | -120.93277777777779 | 91.0 | 299.0 | 7918 | 4.78 | 4.765 | 0.015 | 12.379000000000001 | 12.341 | 0.039 | 0.31 |
193 | Irvine | 33.68416666666666 | -117.7925 | 17.0 | 45.0 | 215529 | 66.454 | 66.10600000000001 | 0.348 | 172.115 | 171.21400000000003 | 0.9009999999999999 | 0.52 |
194 | Irwindale | 34.11666666666667 | -117.96666666666667 | 143.0 | 469.0 | 1422 | 9.613 | 8.826 | 0.787 | 24.897 | 22.859 | 2.0380000000000003 | 8.19 |
195 | Isleton | 38.161944444444444 | -121.60916666666667 | 3.0 | 10.0 | 804 | 0.49200000000000005 | 0.44 | 0.052000000000000005 | 1.272 | 1.139 | 0.134 | 10.5 |
196 | Jackson | 38.348888888888894 | -120.77416666666666 | 371.0 | 1217.0 | 4651 | 3.73 | 3.73 | 0.0 | 9.66 | 9.66 | 0.0 | 0.0 |
197 | JurupaValley | 33.99944444444444 | -117.47527777777778 | 97496 | 43.5 | 43.3 | 0.2 | 112.7 | 112.1 | 0.6 | |||
198 | Kerman | 36.72361111111111 | -120.06 | 67.0 | 220.0 | 17303 | 3.233 | 3.233 | 0.0 | 8.372 | 8.372 | 0.0 | 0.0 |
199 | KingCity | 36.21277777777778 | -121.1261111111111 | 102.0 | 335.0 | 12874 | 3.984 | 3.845 | 0.139 | 10.317 | 9.958 | 0.36 | 3.49 |
200 | Kingsburg | 36.513888888888886 | -119.55388888888889 | 92.0 | 302.0 | 11382 | 2.8280000000000003 | 2.8280000000000003 | 0.0 | 7.325 | 7.325 | 0.0 | 0.0 |
201 | LaCaadaFlintridge | 34.207721 | -118.206979 | 20246 | 8.645 | 8.628 | 0.017 | 22.391 | 22.346999999999998 | 0.044000000000000004 | 0.2 | ||
202 | Lafayette | 37.88583333333333 | -122.11805555555554 | 97.5 | 320.0 | 24285 | 15.387 | 15.220999999999998 | 0.166 | 39.853 | 39.422 | 0.431 | 1.08 |
203 | LagunaBeach | 33.53138888888889 | -117.76916666666666 | 6.0 | 20.0 | 22723 | 9.821 | 8.85 | 0.971 | 25.435 | 22.921 | 2.515 | 9.89 |
204 | LagunaHills | 33.599722222222226 | -117.69944444444445 | 113.0 | 371.0 | 30344 | 6.695 | 6.67 | 0.025 | 17.34 | 17.276 | 0.064 | 0.37 |
205 | LagunaNiguel | 33.53194444444444 | -117.7025 | 121.0 | 397.0 | 62979 | 14.885 | 14.833 | 0.052000000000000005 | 38.551 | 38.418 | 0.134 | 0.35 |
206 | LagunaWoods | 33.60916666666667 | -117.73277777777778 | 116.0 | 381.0 | 18747 | 3.115 | 3.115 | 0.0 | 8.07 | 8.068999999999999 | 0.0 | 0.01 |
207 | LaHabra | 33.93194444444444 | -117.94611111111111 | 91.0 | 299.0 | 60239 | 7.376 | 7.37 | 0.006 | 19.104 | 19.088 | 0.016 | 0.08 |
208 | LaHabraHeights | 33.96388888888889 | -117.95277777777778 | 225.0 | 738.0 | 5325 | 6.162000000000001 | 6.159 | 0.003 | 15.958 | 15.95 | 0.006999999999999999 | 0.05 |
209 | LakeElsinore | 33.68138888888889 | -117.34527777777777 | 395.0 | 1306.0 | 51821 | 41.687 | 36.208 | 5.479 | 107.97 | 93.779 | 14.19 | 13.14 |
210 | LakeForest | 33.641666666666666 | -117.69083333333333 | 148.0 | 400.0 | 77264 | 17.902 | 17.816 | 0.086 | 46.36600000000001 | 46.143 | 0.223 | 0.48 |
211 | Lakeport | 39.043055555555554 | -122.91583333333334 | 413.0 | 1355.0 | 4753 | 3.198 | 3.0580000000000003 | 0.14 | 8.283999999999999 | 7.92 | 0.364 | 4.39 |
212 | Lakewood | 33.847500000000004 | -118.11999999999999 | 14.0 | 46.0 | 80048 | 9.466000000000001 | 9.415 | 0.051 | 24.517 | 24.384 | 0.133 | 0.54 |
213 | LaMesa | 32.771388888888886 | -117.02277777777778 | 161.0 | 528.0 | 57065 | 9.116 | 9.076 | 0.04 | 23.612 | 23.506999999999998 | 0.10400000000000001 | 0.44 |
214 | LaMirada | 33.90222222222222 | -118.00972222222222 | 59.0 | 194.0 | 48527 | 7.857 | 7.84 | 0.017 | 20.351 | 20.305999999999997 | 0.045 | 0.22 |
215 | Lancaster | 34.68333333333333 | -118.15 | 719.0 | 2359.0 | 156633 | 94.54700000000001 | 94.27600000000001 | 0.271 | 244.87599999999998 | 244.175 | 0.701 | 0.29 |
216 | LaPalma | 33.849444444444444 | -118.04388888888889 | 14.0 | 46.0 | 15568 | 1.8319999999999999 | 1.808 | 0.024 | 4.744 | 4.6819999999999995 | 0.063 | 1.32 |
217 | LaPuente | 34.0325 | -117.95527777777778 | 107.0 | 351.0 | 39816 | 3.48 | 3.4789999999999996 | 0.001 | 9.012 | 9.01 | 0.002 | 0.02 |
218 | LaQuinta | 33.67527777777777 | -116.2975 | 0.0 | 37467 | 35.551 | 35.117 | 0.434 | 92.07799999999999 | 90.954 | 1.124 | 1.22 | |
219 | Larkspur | 37.93416666666666 | -122.53527777777778 | 43.0 | 11926 | 3.2430000000000003 | 3.0269999999999997 | 0.21600000000000003 | 8.399 | 7.84 | 0.56 | 6.66 | |
220 | Lathrop | 37.816944444444445 | -121.28861111111111 | 7.0 | 20.0 | 18023 | 23.033 | 21.930999999999997 | 1.102 | 59.655 | 56.8 | 2.8539999999999996 | 4.79 |
221 | LaVerne | 34.114444444444445 | -117.7713888888889 | 323.0 | 1060.0 | 31063 | 8.562000000000001 | 8.43 | 0.132 | 22.175 | 21.834 | 0.341 | 1.54 |
222 | Lawndale | 33.88666666666666 | -118.3536111111111 | 18.0 | 59.0 | 32769 | 1.974 | 1.974 | 0.0 | 5.113 | 5.113 | 0.0 | 0.0 |
223 | LemonGrove | 32.74138888888889 | -117.03166666666667 | 136.0 | 440.0 | 25320 | 3.88 | 3.88 | 0.0 | 10.05 | 10.05 | 0.0 | 0.0 |
224 | Lemoore | 36.30083333333333 | -119.78277777777778 | 70.0 | 230.0 | 25281 | 8.517000000000001 | 8.517000000000001 | 0.0 | 22.058000000000003 | 22.058000000000003 | 0.0 | 0.0 |
225 | Lincoln | 38.88722222222222 | -121.2961111111111 | 51.0 | 167.0 | 42819 | 20.13 | 20.105999999999998 | 0.024 | 52.137 | 52.075 | 0.062 | 0.12 |
226 | Lindsay | 36.2 | -119.08333333333333 | 118.0 | 387.0 | 11768 | 2.61 | 2.61 | 0.0 | 6.7589999999999995 | 6.7589999999999995 | 0.0 | 0.0 |
227 | LiveOakSutterCounty | 39.27583333333333 | -121.66000000000001 | 79.0 | 8392 | 1.869 | 1.869 | 0.0 | 4.8389999999999995 | 4.8389999999999995 | 0.0 | 0.0 | |
228 | Livermore | 37.68194444444444 | -121.76805555555555 | 495.0 | 83547 | 25.176 | 25.173000000000002 | 0.003 | 65.204 | 65.19800000000001 | 0.006999999999999999 | 0.01 | |
229 | Livingston | 37.386944444444445 | -120.72361111111111 | 40.0 | 131.0 | 13058 | 3.715 | 3.715 | 0.0 | 9.622 | 9.622 | 0.0 | 0.0 |
230 | Lodi | 38.12888888888889 | -121.28083333333333 | 15.0 | 50.0 | 62134 | 13.825 | 13.610999999999999 | 0.214 | 35.805 | 35.251999999999995 | 0.5529999999999999 | 1.54 |
231 | LomaLinda | 34.04833333333333 | -117.25055555555555 | 355.0 | 1165.0 | 23261 | 7.517 | 7.516 | 0.001 | 19.47 | 19.467 | 0.002 | 0.01 |
232 | Lomita | 33.79333333333333 | -118.31611111111111 | 29.0 | 95.0 | 20256 | 1.911 | 1.911 | 0.0 | 4.949 | 4.949 | 0.0 | 0.0 |
233 | Lompoc | 34.64611111111111 | -120.46027777777778 | 32.0 | 105.0 | 42434 | 11.675 | 11.597000000000001 | 0.078 | 30.237 | 30.037 | 0.201 | 0.66 |
234 | LongBeach | 33.76833333333333 | -118.19555555555556 | 0.0 | 462257 | 51.437 | 50.293 | 1.1440000000000001 | 133.22299999999998 | 130.259 | 2.964 | 2.22 | |
235 | Loomis | 38.81638888888889 | -121.19277777777778 | 123.0 | 404.0 | 6430 | 7.267 | 7.267 | 0.0 | 18.822 | 18.822 | 0.0 | 0.0 |
236 | LosAlamitos | 33.80222222222222 | -118.06444444444445 | 7.0 | 23.0 | 11449 | 4.1160000000000005 | 4.05 | 0.066 | 10.659 | 10.489 | 0.17 | 1.6 |
237 | LosAltos | 37.36805555555556 | -122.0975 | 157.0 | 28976 | 6.487 | 6.487 | 0.0 | |||||
238 | LosAltosHills | 37.37138888888889 | -122.1375 | 89.0 | 292.0 | 7922 | 8.802 | 8.802 | 0.0 | 22.796999999999997 | 22.796999999999997 | 0.0 | 0.0 |
239 | LosAngeles | 34.05 | -118.25 | 71.0 | 2.0 | 3884307 | 503.0 | 469.0 | 34.0 | 1302.0 | 1214.0 | 88.0 | 6.7 |
240 | LosBanos | 37.05833333333333 | -120.85 | 36.0 | 118.0 | 35972 | 10.117 | 9.993 | 0.124 | 26.203000000000003 | 25.881999999999998 | 0.321 | 1.22 |
241 | LosGatos | 37.236111111111114 | -121.96166666666667 | 105.0 | 344.0 | 29413 | 11.16 | 11.08 | 0.08 | 28.903000000000002 | 28.697 | 0.20600000000000002 | 0.71 |
242 | Loyalton | 39.67666666666666 | -120.24305555555556 | 1509.0 | 4951.0 | 769 | 0.355 | 0.355 | 0.0 | 0.9209999999999999 | 0.9209999999999999 | 0.0 | 0.0 |
243 | Lynwood | 33.92472222222222 | -118.20194444444445 | 28.0 | 92.0 | 69772 | 4.84 | 4.84 | 0.0 | 12.536 | 12.536 | 0.0 | 0.0 |
244 | Madera | 36.96138888888889 | -120.06083333333333 | 83.0 | 271.0 | 61416 | 15.789000000000001 | 15.789000000000001 | 0.0 | 40.894 | 40.894 | 0.0 | 0.0 |
245 | Malibu | 34.03 | -118.75 | 32.0 | 105.0 | 12645 | 19.828 | 19.785 | 0.043 | 51.354 | 51.242 | 0.113 | 0.22 |
246 | MammothLakes | 37.64861111111111 | -118.97194444444445 | 2402.0 | 7880.0 | 8234 | 25.305999999999997 | 24.866 | 0.44 | 65.541 | 64.402 | 1.139 | 1.74 |
247 | ManhattanBeach | 33.888888888888886 | -118.40527777777778 | 20.0 | 67.0 | 35135 | 3.9410000000000003 | 3.937 | 0.004 | 10.208 | 10.197000000000001 | 0.01 | 0.1 |
248 | Manteca | 37.80277777777778 | -121.22083333333333 | 11.0 | 38.0 | 71067 | 17.757 | 17.733 | 0.024 | 45.99100000000001 | 45.928999999999995 | 0.062 | 0.13 |
249 | Maricopa | 35.05888888888889 | -119.40083333333334 | 269.0 | 883.0 | 1154 | 1.5019999999999998 | 1.5019999999999998 | 0.0 | 3.89 | 3.89 | 0.0 | 0.0 |
250 | Marina | 36.68444444444444 | -121.80222222222221 | 13.0 | 43.0 | 29718 | 9.763 | 8.883 | 0.88 | 25.287 | 23.006999999999998 | 2.28 | 9.02 |
251 | Martinez | 38.019444444444446 | -122.13416666666667 | 7.0 | 23.0 | 35824 | 13.135 | 12.130999999999998 | 1.004 | 34.019 | 31.42 | 2.6 | 7.64 |
252 | Marysville | 39.15 | -121.58333333333333 | 19.0 | 62.0 | 12072 | 3.585 | 3.464 | 0.121 | 9.283999999999999 | 8.971 | 0.312 | 3.36 |
253 | Maywood | 33.98777777777778 | -118.18666666666667 | 46.0 | 151.0 | 27395 | 1.178 | 1.178 | 0.0 | 3.052 | 3.052 | 0.0 | 0.0 |
254 | McFarland | 35.67805555555555 | -119.22916666666667 | 108.0 | 354.0 | 13745 | 2.668 | 2.668 | 0.0 | 6.91 | 6.91 | 0.0 | 0.0 |
255 | Mendota | 36.75361111111111 | -120.38166666666666 | 53.0 | 174.0 | 11014 | 3.281 | 3.278 | 0.003 | 8.499 | 8.491 | 0.008 | 0.1 |
256 | Menifee | 33.67833333333333 | -117.16694444444445 | 434.0 | 1424.0 | 77519 | 46.607 | 46.466 | 0.141 | 120.711 | 120.345 | 0.365 | 0.3 |
257 | MenloPark | 37.45277777777778 | -122.18333333333334 | 22.0 | 72.0 | 32026 | 17.415 | 9.79 | 7.625 | 45.105 | 25.355 | 19.75 | 43.79 |
258 | Merced | 37.3 | -120.48333333333333 | 52.0 | 171.0 | 80793 | 23.316 | 23.316 | 0.0 | 60.388999999999996 | 60.388999999999996 | 0.0 | 0.0 |
259 | Millbrae | 37.600833333333334 | -122.40138888888889 | 33.0 | 21536 | 3.259 | 3.247 | 0.012 | 8.439 | 8.408999999999999 | 0.03 | 0.36 | |
260 | MillValley | 37.90611111111111 | -122.545 | 79.0 | 13903 | 4.8469999999999995 | 4.763 | 0.084 | 12.555 | 12.335999999999999 | 0.21899999999999997 | 1.74 | |
261 | Milpitas | 37.43472222222222 | -121.89500000000001 | 5.0 | 16.0 | 70092 | 13.640999999999998 | 13.591 | 0.05 | 35.328 | 35.2 | 0.128 | 0.36 |
262 | MissionViejo | 33.61277777777778 | -117.65611111111112 | 121.0 | 448.0 | 96346 | 18.123 | 17.739 | 0.384 | 46.93899999999999 | 45.943999999999996 | 0.995 | 2.12 |
263 | Modesto | 37.66138888888889 | -120.99444444444444 | 27.0 | 89.0 | 201165 | 37.092 | 36.867 | 0.225 | 96.069 | 95.486 | 0.583 | 0.61 |
264 | Monrovia | 34.14416666666666 | -118.00194444444445 | 174.0 | 571.0 | 36590 | 13.714 | 13.605 | 0.109 | 35.519 | 35.236999999999995 | 0.282 | 0.79 |
265 | Montague | 41.727222222222224 | -122.52638888888889 | 774.0 | 2539.0 | 1443 | 1.7930000000000001 | 1.778 | 0.015 | 4.644 | 4.605 | 0.039 | 0.85 |
266 | Montclair | 34.07083333333334 | -117.6975 | 326.0 | 1066.0 | 36664 | 5.517 | 5.517 | 0.0 | 14.289000000000001 | 14.289000000000001 | 0.0 | 0.0 |
267 | Montebello | 34.01444444444444 | -118.11444444444444 | 61.0 | 200.0 | 62500 | 8.373 | 8.333 | 0.04 | 21.685 | 21.581 | 0.10400000000000001 | 0.48 |
268 | Monterey | 36.6 | -121.9 | 8.0 | 26.0 | 27810 | 11.764000000000001 | 8.466000000000001 | 3.298 | 30.469 | 21.927 | 8.542 | 28.03 |
269 | MontereyPark | 34.049166666666665 | -118.13555555555556 | 117.0 | 384.0 | 60269 | 7.733 | 7.672000000000001 | 0.061 | 20.029 | 19.87 | 0.158 | 0.79 |
270 | MonteSereno | 37.238055555555555 | -121.98944444444444 | 515.0 | 3341 | 1.615 | 1.615 | 0.0 | 4.184 | 4.184 | 0.0 | 0.0 | |
271 | Moorpark | 34.280833333333334 | -118.87333333333332 | 157.0 | 515.0 | 34421 | 12.799000000000001 | 12.579 | 0.22 | 33.149 | 32.58 | 0.569 | 1.72 |
272 | Moraga | 37.835 | -122.12972222222221 | 16016 | 9.442 | 9.433 | 0.009000000000000001 | 24.455 | 24.432 | 0.023 | 0.09 | ||
273 | MorenoValley | 33.94305555555555 | -117.22833333333334 | 497.0 | 1631.0 | 193365 | 51.475 | 51.275 | 0.2 | 133.319 | 132.8 | 0.519 | 0.39 |
274 | MorganHill | 37.13055555555555 | -121.65444444444445 | 107.0 | 350.0 | 37882 | 12.882 | 12.882 | 0.0 | 33.363 | 33.363 | 0.0 | 0.0 |
275 | MorroBay | 35.37916666666667 | -120.85333333333332 | 19.0 | 62.0 | 10234 | 10.322000000000001 | 5.303 | 5.019 | 26.734 | 13.734000000000002 | 13.0 | 48.63 |
276 | MountainView | 37.38944444444444 | -122.08194444444445 | 32.0 | 105.0 | 74066 | 12.273 | 11.995 | 0.278 | 31.788 | 31.068 | 0.72 | 2.26 |
277 | MountShasta | 41.31444444444444 | -122.31138888888889 | 1099.0 | 3606.0 | 3394 | 3.77 | 3.766 | 0.004 | 9.764 | 9.754 | 0.01 | 0.1 |
278 | Murrieta | 33.56944444444445 | -117.2025 | 334.0 | 1096.0 | 103466 | 33.613 | 33.577 | 0.036000000000000004 | 87.05799999999999 | 86.964 | 0.094 | 0.11 |
279 | Napa | 38.30472222222222 | -122.29888888888888 | 20.0 | 76915 | 18.147000000000002 | 17.839000000000002 | 0.308 | 47.0 | 46.203 | 0.797 | 1.69 | |
280 | NationalCity | 32.67805555555555 | -117.09916666666666 | 21.0 | 69.0 | 58582 | 9.116 | 7.277 | 1.839 | 23.609 | 18.847 | 4.762 | 20.17 |
281 | Needles | 34.83833333333334 | -114.6111111111111 | 151.0 | 495.0 | 4844 | 31.275 | 30.808000000000003 | 0.467 | 81.002 | 79.793 | 1.209 | 1.49 |
282 | NevadaCity | 39.26138888888889 | -121.01861111111111 | 755.0 | 2477.0 | 3068 | 2.1919999999999997 | 2.188 | 0.004 | 5.6770000000000005 | 5.667000000000001 | 0.01 | 0.17 |
283 | Newark | 37.53333333333333 | -122.03333333333333 | 20.0 | 43539 | 13.898 | 13.875 | 0.023 | 35.996 | 35.936 | 0.061 | 0.17 | |
284 | Newman | 37.315 | -121.0225 | 27.0 | 89.0 | 10224 | 2.102 | 2.102 | 0.0 | 5.444 | 5.444 | 0.0 | 0.0 |
285 | NewportBeach | 33.61666666666667 | -117.89750000000001 | 3.0 | 85186 | 52.978 | 23.805 | 29.173000000000002 | 137.211 | 61.653999999999996 | 75.557 | 55.07 | |
286 | Norco | 33.93111111111111 | -117.54861111111111 | 195.0 | 640.0 | 27063 | 14.277999999999999 | 13.962 | 0.316 | 36.98 | 36.161 | 0.8190000000000001 | 2.22 |
287 | Norwalk | 33.90694444444444 | -118.08333333333333 | 28.0 | 92.0 | 105549 | 9.746 | 9.707 | 0.039 | 25.243000000000002 | 25.141 | 0.102 | 0.4 |
288 | Novato | 38.1075 | -122.56972222222223 | 9.0 | 30.0 | 51904 | 27.956999999999997 | 27.44 | 0.517 | 72.407 | 71.068 | 1.339 | 1.85 |
289 | Oakdale | 37.76916666666666 | -120.85694444444444 | 48.0 | 157.0 | 20675 | 6.095 | 6.045 | 0.05 | 15.784 | 15.655 | 0.129 | 0.81 |
290 | Oakland | 37.80444444444444 | -122.27083333333333 | 43.0 | 406253 | 78.002 | 55.786 | 22.215999999999998 | 202.024 | 144.485 | 57.54 | 28.48 | |
291 | Oakley | 37.9975 | -121.7125 | 6.0 | 20.0 | 35432 | 16.155 | 15.853 | 0.302 | 41.842 | 41.059 | 0.7829999999999999 | 1.87 |
292 | Oceanside | 33.211666666666666 | -117.32583333333334 | 20.0 | 66.0 | 183095 | 42.174 | 41.235 | 0.9390000000000001 | 109.23100000000001 | 106.79799999999999 | 2.4330000000000003 | 2.23 |
293 | Ojai | 34.44916666666666 | -119.24666666666667 | 227.0 | 745.0 | 7461 | 4.401 | 4.386 | 0.015 | 11.398 | 11.359000000000002 | 0.04 | 0.35 |
294 | Ontario | 34.05277777777778 | -117.62777777777777 | 282.0 | 925.0 | 163924 | 50.006 | 49.941 | 0.065 | 129.515 | 129.345 | 0.17 | 0.13 |
295 | Orange | 33.80305555555555 | -117.8325 | 59.0 | 195.0 | 136416 | 25.24 | 24.796999999999997 | 0.44299999999999995 | 65.37100000000001 | 64.22399999999999 | 1.147 | 1.75 |
296 | OrangeCove | 36.62444444444444 | -119.31361111111111 | 129.0 | 423.0 | 9078 | 1.912 | 1.912 | 0.0 | 4.9510000000000005 | 4.9510000000000005 | 0.0 | 0.0 |
297 | Orinda | 37.882777777777775 | -122.17972222222222 | 151.0 | 495.0 | 18342 | 12.698 | 12.683 | 0.015 | 32.887 | 32.848 | 0.039 | 0.12 |
298 | Orland | 39.7475 | -122.19638888888889 | 79.0 | 259.0 | 7291 | 2.971 | 2.971 | 0.0 | 7.696000000000001 | 7.696000000000001 | 0.0 | 0.0 |
299 | Oroville | 39.516666666666666 | -121.55 | 51.0 | 167.0 | 15506 | 13.011 | 12.993 | 0.018000000000000002 | 33.701 | 33.653 | 0.048 | 0.14 |
300 | Oxnard | 34.19138888888889 | -119.1825 | 16.0 | 52.0 | 203585 | 39.208 | 26.894000000000002 | 12.314 | 101.54799999999999 | 69.656 | 31.893 | 31.41 |
301 | Pacifica | 37.62277777777778 | -122.48555555555555 | 25.0 | 82.0 | 37234 | 12.66 | 12.658 | 0.002 | 32.789 | 32.784 | 0.005 | 0.01 |
302 | PacificGrove | 36.617777777777775 | -121.91666666666667 | 46.0 | 151.0 | 15295 | 4.003 | 2.865 | 1.138 | 10.366 | 7.419 | 2.946 | 28.42 |
303 | Palmdale | 34.58111111111111 | -118.10055555555554 | 810.0 | 2657.0 | 152750 | 106.21600000000001 | 105.961 | 0.255 | 275.099 | 274.439 | 0.66 | 0.24 |
304 | PalmDesert | 33.72555555555556 | -116.36944444444444 | 67.0 | 220.0 | 48445 | 27.014 | 26.81 | 0.204 | 69.96600000000001 | 69.437 | 0.529 | 0.76 |
305 | PalmSprings | 33.823888888888895 | -116.53027777777778 | 146.0 | 440.0 | 44552 | 94.975 | 94.116 | 0.8590000000000001 | 245.984 | 243.761 | 2.224 | 0.9 |
306 | PaloAlto | 37.42916666666667 | -122.13805555555557 | 9.0 | 30.0 | 64403 | 25.787 | 23.884 | 1.903 | 66.78699999999999 | 61.858000000000004 | 4.928999999999999 | 7.38 |
307 | PalosVerdesEstates | 33.786944444444444 | -118.39666666666668 | 64.0 | 210.0 | 13438 | 4.774 | 4.774 | 0.0 | 12.365 | 12.364 | 0.001 | 0.01 |
308 | Paradise | 39.75972222222222 | -121.62138888888889 | 542.0 | 1778.0 | 26249 | 18.322 | 18.308 | 0.013999999999999999 | 47.455 | 47.418 | 0.037000000000000005 | 0.08 |
309 | Paramount | 33.9 | -118.16666666666667 | 21.0 | 69.0 | 54098 | 4.84 | 4.729 | 0.111 | 12.536 | 12.249 | 0.28600000000000003 | 2.28 |
310 | Parlier | 36.611666666666665 | -119.52694444444444 | 105.0 | 344.0 | 14494 | 2.194 | 2.194 | 0.0 | 5.682 | 5.682 | 0.0 | 0.0 |
311 | Pasadena | 34.15611111111111 | -118.13194444444444 | 263.0 | 863.0 | 137122 | 23.128 | 22.97 | 0.158 | 59.902 | 59.493 | 0.409 | 0.68 |
312 | PasoRobles | 35.64083333333333 | -120.6538888888889 | 242.0 | 29792 | 19.425 | 19.12 | 0.305 | 50.312 | 49.522 | 0.79 | 1.57 | |
313 | Patterson | 37.473055555555554 | -121.13277777777778 | 31.0 | 102.0 | 20413 | 5.954 | 5.954 | 0.0 | 15.421 | 15.421 | 0.0 | 0.0 |
314 | Perris | 33.79666666666667 | -117.22444444444444 | 443.0 | 1453.0 | 71326 | 31.503 | 31.393 | 0.11 | 81.594 | 81.308 | 0.28600000000000003 | 0.35 |
315 | Petaluma | 38.24583333333334 | -122.63138888888888 | 9.0 | 30.0 | 58921 | 14.489 | 14.382 | 0.107 | 37.527 | 37.249 | 0.278 | 0.74 |
316 | PicoRivera | 33.98888888888889 | -118.08916666666666 | 50.0 | 164.0 | 62942 | 8.882 | 8.296 | 0.586 | 23.003 | 21.485 | 1.518 | 6.6 |
317 | Piedmont | 37.81666666666667 | -122.23333333333333 | 331.0 | 10667 | 1.6780000000000002 | 1.6780000000000002 | 0.0 | 4.345 | 4.345 | 0.0 | 0.0 | |
318 | Pinole | 38.004444444444445 | -122.2911111111111 | 31.0 | 23.0 | 18390 | 13.575 | 5.3229999999999995 | 8.252 | 35.16 | 13.787 | 21.372 | 60.79 |
319 | PismoBeach | 35.14833333333333 | -120.64805555555556 | 17.0 | 56.0 | 7655 | 13.475999999999999 | 3.5989999999999998 | 9.877 | 34.904 | 9.322000000000001 | 25.581999999999997 | 73.29 |
320 | Pittsburg | 38.028055555555554 | -121.88472222222222 | 8.0 | 26.0 | 63264 | 19.154 | 17.218 | 1.936 | 49.61 | 44.595 | 5.015 | 10.11 |
321 | Placentia | 33.8825 | -117.85499999999999 | 83.0 | 272.0 | 50533 | 6.582000000000001 | 6.568 | 0.013999999999999999 | 17.048 | 17.011 | 0.037000000000000005 | 0.22 |
322 | Placerville | 38.72972222222222 | -120.79861111111111 | 1867.0 | 10389 | 5.813 | 5.812 | 0.001 | 15.054 | 15.052 | 0.002 | 0.01 | |
323 | PleasantHill | 37.948055555555555 | -122.0525 | 16.0 | 52.0 | 33152 | 7.072 | 7.072 | 0.0 | 18.315 | 18.315 | 0.0 | 0.0 |
324 | Pleasanton | 37.6625 | -121.87472222222222 | 351.0 | 70285 | 24.266 | 24.113000000000003 | 0.153 | 62.847 | 62.452 | 0.395 | 0.63 | |
325 | Plymouth | 38.481944444444444 | -120.84472222222222 | 330.0 | 1083.0 | 1005 | 0.9440000000000001 | 0.9309999999999999 | 0.013000000000000001 | 2.444 | 2.411 | 0.033 | 1.34 |
326 | PointArena | 38.90888888888889 | -123.69305555555556 | 36.0 | 118.0 | 449 | 1.35 | 1.35 | 0.0 | 3.4960000000000004 | 3.4960000000000004 | 0.0 | 0.0 |
327 | Pomona | 34.06083333333333 | -117.75583333333333 | 259.0 | 850.0 | 1 | 22.964000000000002 | 22.951999999999998 | 0.012 | 59.474 | 59.443999999999996 | 0.03 | 0.05 |
328 | Porterville | 36.06861111111112 | -119.0275 | 139.0 | 459.0 | 55697 | 17.679000000000002 | 17.607 | 0.07200000000000001 | 45.79 | 45.603 | 0.188 | 0.41 |
329 | PortHueneme | 34.16027777777778 | -119.19444444444444 | 4.0 | 12.0 | 21723 | 4.671 | 4.4510000000000005 | 0.22 | 12.095999999999998 | 11.527999999999999 | 0.569 | 4.7 |
330 | Portola | 39.81027777777778 | -120.46972222222223 | 1480.0 | 4856.0 | 2104 | 5.407 | 5.407 | 0.0 | 14.003 | 14.003 | 0.0 | 0.0 |
331 | PortolaValley | 37.375 | -122.21861111111112 | 140.0 | 459.0 | 4353 | 9.093 | 9.092 | 0.001 | 23.551 | 23.546999999999997 | 0.004 | 0.02 |
332 | Poway | 32.97 | -117.03861111111111 | 157.0 | 515.0 | 47811 | 39.165 | 39.079 | 0.086 | 101.43799999999999 | 101.214 | 0.223 | 0.22 |
333 | RanchoCordova | 38.58916666666667 | -121.30277777777778 | 27.0 | 89.0 | 64776 | 33.874 | 33.507 | 0.36700000000000005 | 87.73299999999999 | 86.78200000000001 | 0.951 | 1.08 |
334 | RanchoCucamonga | 34.123333333333335 | -117.57944444444443 | 368.0 | 1207.0 | 170746 | 39.871 | 39.851 | 0.02 | 103.26299999999999 | 103.212 | 0.051 | 0.05 |
335 | RanchoMirage | 33.76916666666666 | -116.42111111111112 | 83.0 | 272.0 | 17218 | 24.447 | 0.389 | 64.32600000000001 | 63.318000000000005 | 1.008 | 1.57 | |
336 | RanchoPalosVerdes | 33.75833333333333 | -118.36416666666666 | 67.0 | 220.0 | 41643 | 13.465 | 13.465 | 0.0 | 34.875 | 34.874 | 0.001 | 0.0 |
337 | RanchoSantaMargarita | 33.64138888888889 | -117.59444444444443 | 290.0 | 775.0 | 47853 | 12.992 | 12.957 | 0.035 | 33.650999999999996 | 33.56 | 0.091 | 0.27 |
338 | RedBluff | 40.17666666666666 | -122.23805555555556 | 93.0 | 305.0 | 24674 | 7.6770000000000005 | 7.563 | 0.114 | 19.882 | 19.587 | 0.295 | 1.48 |
339 | Redding | 40.583333333333336 | -122.36666666666666 | 151.0 | 495.0 | 92328 | 61.175 | 59.647 | 1.528 | 158.442 | 154.485 | 3.957 | 2.5 |
340 | Redlands | 34.05472222222222 | -117.1825 | 414.0 | 1358.0 | 68747 | 36.427 | 36.126 | 0.301 | 94.344 | 93.565 | 0.779 | 0.83 |
341 | RedondoBeach | 33.85638888888889 | -118.37694444444443 | 62.0 | 66748 | 6.207999999999999 | 6.1979999999999995 | 0.01 | 16.08 | 16.054000000000002 | 0.026000000000000002 | 0.16 | |
342 | RedwoodCity | 37.48277777777778 | -122.23611111111111 | 6.0 | 20.0 | 76815 | 34.625 | 19.42 | 15.205 | 89.677 | 50.297 | 39.38 | 43.91 |
343 | Reedley | 36.59638888888889 | -119.45027777777779 | 106.0 | 348.0 | 24194 | 5.156000000000001 | 5.084 | 0.07200000000000001 | 13.352 | 13.165999999999999 | 0.185 | 1.39 |
344 | Rialto | 34.11138888888889 | -117.3825 | 383.0 | 1257.0 | 101740 | 22.365 | 22.351 | 0.013999999999999999 | 57.926 | 57.888999999999996 | 0.037000000000000005 | 0.06 |
345 | Richmond | 37.93583333333333 | -122.34777777777778 | 14.0 | 46.0 | 103701 | 52.48 | 30.068 | 22.412 | 135.923 | 77.875 | 58.048 | 42.71 |
346 | Ridgecrest | 35.6225 | -117.67083333333333 | 698.0 | 2290.0 | 27616 | 21.416999999999998 | 20.766 | 0.6509999999999999 | 55.468999999999994 | 53.783 | 1.685 | 3.04 |
347 | RioDell | 40.49916666666667 | -124.10638888888889 | 49.0 | 161.0 | 3363 | 2.418 | 2.282 | 0.136 | 6.261 | 5.91 | 0.35100000000000003 | 5.61 |
348 | RioVista | 38.163888888888884 | -121.69583333333334 | 6.0 | 20.0 | 7360 | 7.093999999999999 | 6.691 | 0.40299999999999997 | 18.375 | 17.33 | 1.044 | 5.68 |
349 | Ripon | 37.74055555555556 | -121.12833333333333 | 21.0 | 69.0 | 14297 | 5.495 | 5.305 | 0.19 | 14.232000000000001 | 13.739 | 0.493 | 3.47 |
350 | Riverbank | 37.731388888888894 | -120.94361111111111 | 43.0 | 141.0 | 22678 | 4.1160000000000005 | 4.092 | 0.024 | 10.661 | 10.599 | 0.062 | 0.59 |
351 | Riverside | 33.948055555555555 | -117.39611111111111 | 262.0 | 860.0 | 313673 | 81.444 | 81.14 | 0.304 | 210.94099999999997 | 210.15200000000002 | 0.7879999999999999 | 0.37 |
352 | Rocklin | 38.8 | -121.24666666666667 | 79.0 | 249.0 | 56974 | 19.594 | 19.541 | 0.053 | 50.748999999999995 | 50.61 | 0.139 | 0.27 |
353 | RohnertPark | 38.34722222222222 | -122.69527777777778 | 32.0 | 105.0 | 40971 | 7.007999999999999 | 7.002999999999999 | 0.005 | 18.149 | 18.136 | 0.013000000000000001 | 0.07 |
354 | RollingHills | 33.75944444444445 | -118.34166666666667 | 389.0 | 1276.0 | 1860 | 2.991 | 2.991 | 0.0 | 7.746 | 7.746 | 0.0 | 0.0 |
355 | RollingHillsEstates | 33.77361111111111 | -118.36083333333333 | 143.0 | 469.0 | 8067 | 3.613 | 3.569 | 0.044000000000000004 | 9.359 | 9.244 | 0.115 | 1.22 |
356 | Rosemead | 34.06666666666667 | -118.08333333333333 | 97.0 | 318.0 | 53764 | 5.176 | 5.162000000000001 | 0.013999999999999999 | 13.405999999999999 | 13.37 | 0.035 | 0.26 |
357 | Roseville | 38.7525 | -121.28944444444444 | 50.0 | 164.0 | 127323 | 36.223 | 36.222 | 0.001 | 93.81700000000001 | 93.814 | 0.003 | 0.0 |
358 | Ross | 37.962500000000006 | -122.55499999999999 | 11.0 | 36.0 | 2415 | 1.556 | 1.556 | 0.0 | 4.031000000000001 | 4.031000000000001 | 0.0 | 0.0 |
359 | Sacramento | 38.55555555555555 | -121.46888888888888 | 9.0 | 30.0 | 466488 | 100.105 | 97.915 | 2.19 | 259.27299999999997 | 253.6 | 5.672999999999999 | 2.19 |
360 | Salinas | 36.67777777777778 | -121.65555555555557 | 16.0 | 52.0 | 163665 | 23.217 | 23.179000000000002 | 0.038 | 60.131 | 60.033 | 0.099 | 0.16 |
361 | SanAnselmo | 37.974722222222226 | -122.56166666666667 | 14.0 | 46.0 | 12336 | 2.677 | 2.677 | 0.0 | 6.934 | 6.934 | 0.0 | 0.0 |
362 | SanBernardino | 34.1 | -117.3 | 321.0 | 1974.0 | 209924 | 59.645 | 59.201 | 0.444 | 154.48 | 153.33 | 1.15 | 0.74 |
363 | SanBruno | 37.625277777777775 | -122.42527777777778 | 5.0 | 16.0 | 41114 | 5.478 | 5.478 | 0.0 | 14.187999999999999 | 14.187999999999999 | 0.0 | 0.0 |
364 | SanCarlos | 37.49916666666667 | -122.26333333333334 | 33.0 | 28406 | 5.541 | 5.537999999999999 | 0.003 | 14.35 | 14.343 | 0.006999999999999999 | 0.05 | |
365 | SanClemente | 33.437777777777775 | -117.62027777777777 | 71.0 | 250.0 | 63522 | 19.468 | 18.711 | 0.757 | 50.422 | 48.461000000000006 | 1.9609999999999999 | 3.89 |
366 | SandCity | 36.617222222222225 | -121.84833333333333 | 22.0 | 72.0 | 334 | 2.924 | 0.562 | 2.362 | 7.575 | 1.4569999999999999 | 6.119 | 80.77 |
367 | SanDiego | 32.715 | -117.16250000000001 | 6.0 | 3.0 | 1345895 | 372.4 | 325.19 | 47.21 | 964.51 | 842.23 | 122.27 | 12.68 |
368 | SanDimas | 34.10277777777778 | -117.81611111111111 | 750.0 | 33371 | 15.427 | 15.037 | 0.39 | 39.957 | 38.946999999999996 | 1.01 | 2.53 | |
369 | SanFernando | 34.28722222222222 | -118.4388888888889 | 326.0 | 1070.0 | 23645 | 2.374 | 2.374 | 0.0 | 6.149 | 6.149 | 0.0 | 0.0 |
370 | SanFrancisco | 37.78333333333333 | -122.41666666666667 | 52.0 | 837442 | 231.89 | 46.87 | 185.02 | 80.0 | ||||
371 | SanGabriel | 34.094166666666666 | -118.09833333333333 | 128.0 | 420.0 | 39718 | 4.146 | 4.145 | 0.001 | 10.735999999999999 | 10.734000000000002 | 0.002 | 0.02 |
372 | Sanger | 36.70805555555556 | -119.55583333333333 | 371.0 | 24270 | 5.524 | 5.524 | 0.0 | 14.307 | 14.307 | 0.0 | 0.0 | |
373 | SanJacinto | 33.78722222222222 | -116.96666666666667 | 477.0 | 1565.0 | 44199 | 26.131 | 25.715999999999998 | 0.415 | 67.679 | 66.605 | 1.074 | 1.59 |
374 | SanJoaquin | 36.60666666666667 | -120.18916666666667 | 53.0 | 174.0 | 4001 | 1.148 | 1.148 | 0.0 | 2.9730000000000003 | 2.9730000000000003 | 0.0 | 0.0 |
375 | SanJose | 37.333333333333336 | -121.9 | 26.0 | 1000536 | 179.97 | 176.52599999999998 | 3.4389999999999996 | 466.10900000000004 | 457.20099999999996 | 8.908 | 1.91 | |
376 | SanJuanBautista | 36.844166666666666 | -121.53722222222223 | 66.0 | 217.0 | 1862 | 0.711 | 0.711 | 0.0 | 1.8430000000000002 | 1.8419999999999999 | 0.001 | 0.06 |
377 | SanJuanCapistrano | 33.49944444444444 | -117.66166666666668 | 37.0 | 96.0 | 34593 | 14.295 | 14.115 | 0.18 | 37.024 | 36.559 | 0.466 | 1.26 |
378 | SanLeandro | 37.725 | -122.15611111111112 | 15.0 | 56.0 | 86869 | 15.663 | 13.343 | 2.32 | 40.565 | 34.556999999999995 | 6.007999999999999 | 14.81 |
379 | SanLuisObispo | 35.274166666666666 | -120.66305555555556 | 71.0 | 233.0 | 45119 | 12.93 | 12.777000000000001 | 0.153 | 33.489000000000004 | 33.093 | 0.396 | 1.18 |
380 | SanMarcos | 33.14194444444444 | -117.17027777777778 | 83781 | 24.39 | 24.37 | 0.02 | 63.169 | 63.117 | 0.053 | 0.08 | ||
381 | SanMarino | 34.12277777777778 | -118.11305555555555 | 172.0 | 564.0 | 13147 | 3.7739999999999996 | 3.767 | 0.006999999999999999 | 9.775 | 9.757 | 0.018000000000000002 | 0.18 |
382 | SanMateo | 37.55416666666667 | -122.31305555555555 | 13.0 | 43.0 | 97207 | 15.884 | 12.13 | 3.7539999999999996 | 41.137 | 31.416 | 9.722000000000001 | 23.63 |
383 | SanPablo | 37.96222222222222 | -122.34555555555555 | 16.0 | 52.0 | 29139 | 2.634 | 2.634 | 0.0 | 6.822 | 6.822 | 0.0 | 0.0 |
384 | SanRafael | 37.97361111111111 | -122.53111111111112 | 13.0 | 43.0 | 57713 | 22.421999999999997 | 16.47 | 5.952000000000001 | 58.074 | 42.657 | 15.417 | 26.55 |
385 | SanRamon | 37.78 | -121.97805555555556 | 146.0 | 480.0 | 73927 | 18.077 | 18.061 | 0.016 | 46.818999999999996 | 46.778 | 0.042 | 0.09 |
386 | SantaAna | 33.740833333333335 | -117.88138888888888 | 35.0 | 115.0 | 324528 | 27.518 | 27.27 | 0.248 | 71.271 | 70.628 | 0.643 | 0.9 |
387 | SantaBarbara | 34.42583333333333 | -119.71416666666667 | 15.0 | 49.0 | 90893 | 41.968 | 19.468 | 22.5 | 108.697 | 50.422 | 58.275 | 53.61 |
388 | SantaClara | 37.35444444444445 | -121.96916666666667 | 23.0 | 75.0 | 116468 | 18.407 | 18.407 | 0.0 | 47.675 | 47.675 | 0.0 | 0.0 |
389 | SantaClarita | 34.416666666666664 | -118.50638888888889 | 368.0 | 1207.0 | 209130 | 62.16 | 62.1 | 0.06 | 160.993 | 160.825 | 0.168 | 0.099 |
390 | SantaCruz | 36.971944444444446 | -122.02638888888889 | 11.0 | 36.0 | 62864 | 15.828 | 12.74 | 3.088 | 40.996 | 32.997 | 7.999 | 19.51 |
391 | SantaFeSprings | 33.9375 | -118.06722222222221 | 41.0 | 135.0 | 16223 | 8.914 | 8.874 | 0.04 | 23.088 | 22.985 | 0.10300000000000001 | 0.45 |
392 | SantaMaria | 34.95138888888889 | -120.43333333333334 | 67.0 | 220.0 | 101459 | 23.395 | 22.756 | 0.639 | 60.592 | 58.937 | 1.655 | 2.73 |
393 | SantaMonica | 34.02194444444444 | -118.48138888888889 | 105.0 | 89736 | 8.416 | 8.415 | 0.001 | |||||
394 | SantaPaula | 34.35583333333334 | -119.06833333333333 | 85.0 | 279.0 | 29321 | 4.707 | 4.593 | 0.114 | 12.189 | 11.895 | 0.294 | 2.41 |
395 | SantaRosa | 38.448611111111106 | -122.70472222222223 | 50.0 | 164.0 | 170685 | 41.498999999999995 | 41.294 | 0.205 | 107.48100000000001 | 106.95 | 0.531 | 0.49 |
396 | Santee | 32.86972222222222 | -116.97111111111111 | 105.0 | 345.0 | 53413 | 16.528 | 16.235 | 0.293 | 42.808 | 42.049 | 0.759 | 1.77 |
397 | Saratoga | 37.2725 | -122.01944444444445 | 410.0 | 29926 | 12.382 | 12.382 | 0.0 | 32.07 | 32.07 | 0.0 | 0.0 | |
398 | Sausalito | 37.85916666666667 | -122.48527777777778 | 4.0 | 13.0 | 7061 | 2.2569999999999997 | 1.771 | 0.486 | 5.846 | 4.586 | 1.2590000000000001 | 21.54 |
399 | ScottsValley | 37.05138888888889 | -122.01333333333334 | 171.0 | 561.0 | 11580 | 4.595 | 4.595 | 0.0 | 11.9 | 11.9 | 0.0 | 0.0 |
400 | SealBeach | 33.759166666666665 | -118.0825 | 4.0 | 13.0 | 24168 | 13.04 | 11.286 | 1.754 | 33.775 | 29.230999999999998 | 4.544 | 13.45 |
401 | Seaside | 36.611111111111114 | -121.84472222222222 | 10.0 | 33.0 | 33025 | 9.376 | 9.237 | 0.139 | 24.281999999999996 | 23.923000000000002 | 0.359 | 1.48 |
402 | Sebastopol | 38.399166666666666 | -122.82694444444444 | 25.0 | 82.0 | 7379 | 1.8530000000000002 | 1.8530000000000002 | 0.0 | 4.7989999999999995 | 4.7989999999999995 | 0.0 | 0.0 |
403 | Selma | 36.57083333333334 | -119.61194444444443 | 94.0 | 308.0 | 23219 | 5.136 | 5.136 | 0.0 | 13.302999999999999 | 13.302999999999999 | 0.0 | 0.0 |
404 | Shafter | 35.50055555555556 | -119.27166666666666 | 106.0 | 348.0 | 16988 | 27.945 | 27.945 | 0.0 | 72.376 | 72.376 | 0.0 | 0.0 |
405 | ShastaLake | 40.67805555555555 | -122.36999999999999 | 246.0 | 810.0 | 10164 | 10.929 | 10.921 | 0.008 | 28.305 | 28.284000000000002 | 0.02 | 0.07 |
406 | SierraMadre | 34.164722222222224 | -118.05083333333333 | 252.0 | 827.0 | 10917 | 2.957 | 2.9530000000000003 | 0.004 | 7.659 | 7.647 | 0.012 | 0.15 |
407 | SignalHill | 33.79935 | -118.16558 | 45.0 | 148.0 | 11465 | 2.191 | 2.189 | 0.002 | 5.672999999999999 | 5.669 | 0.004 | 0.08 |
408 | SimiValley | 34.27111111111111 | -118.73944444444444 | 234.0 | 768.0 | 126874 | 42.247 | 41.48 | 0.767 | 109.41799999999999 | 107.43299999999999 | 1.986 | 1.81 |
409 | SolanaBeach | 32.99527777777778 | -117.26027777777777 | 22.0 | 72.0 | 12867 | 3.6239999999999997 | 3.52 | 0.10400000000000001 | 9.386000000000001 | 9.115 | 0.27 | 2.88 |
410 | Soledad | 36.42472222222222 | -121.32638888888889 | 58.0 | 190.0 | 25738 | 4.566 | 4.414 | 0.152 | 11.825 | 11.432 | 0.39299999999999996 | 3.32 |
411 | Solvang | 34.59388888888889 | -120.13972222222223 | 154.0 | 505.0 | 5245 | 2.426 | 2.425 | 0.001 | 6.284 | 6.281000000000001 | 0.003 | 0.05 |
412 | Sonoma | 38.288888888888884 | -122.4588888888889 | 26.0 | 85.0 | 10648 | 2.742 | 2.742 | 0.0 | 7.102 | 7.102 | 0.0 | 0.0 |
413 | Sonora | 37.98444444444444 | -120.38166666666666 | 544.0 | 1785.0 | 7169 | 3.0780000000000003 | 3.0639999999999996 | 0.013999999999999999 | 7.972 | 7.936 | 0.036000000000000004 | 0.45 |
414 | SouthElMonte | 34.04888888888889 | -118.04833333333333 | 76.0 | 249.0 | 20116 | 2.8480000000000003 | 2.843 | 0.005 | 7.377999999999999 | 7.364 | 0.013999999999999999 | 0.19 |
415 | SouthGate | 33.94416666666666 | -118.19500000000001 | 37.0 | 120.0 | 94396 | 7.353 | 7.236000000000001 | 0.11699999999999999 | 19.044 | 18.742 | 0.303 | 1.59 |
416 | SouthLakeTahoe | 38.94 | -119.97694444444444 | 1901.0 | 6237.0 | 21403 | 16.602999999999998 | 10.161 | 6.442 | 43.003 | 26.318 | 16.685 | 38.8 |
417 | SouthPasadena | 34.113055555555555 | -118.15583333333333 | 201.0 | 659.0 | 25619 | 3.417 | 3.405 | 0.012 | 8.851 | 8.82 | 0.031 | 0.35 |
418 | SouthSanFrancisco | 37.65611111111111 | -122.42555555555556 | 4.0 | 13.0 | 64409 | 30.158 | 9.141 | 21.017 | 78.109 | 23.674 | 54.435 | 69.69 |
419 | Stanton | 33.802499999999995 | -117.99444444444444 | 20.0 | 66.0 | 38186 | 3.15 | 3.15 | 0.0 | 8.158 | 8.158 | 0.0 | 0.0 |
420 | StHelena | 38.50527777777778 | -122.47027777777778 | 77.0 | 253.0 | 5814 | 5.027 | 4.986000000000001 | 0.040999999999999995 | 13.019 | 12.913 | 0.106 | 0.81 |
421 | Stockton | 37.97555555555556 | -121.30083333333333 | 4.0 | 13.0 | 301090 | 64.753 | 61.67 | 3.083 | 167.708 | 159.72299999999998 | 7.985 | 4.76 |
422 | SuisunCity | 38.245 | -122.01694444444445 | 2.0 | 7.0 | 28111 | 4.163 | 4.105 | 0.057999999999999996 | 10.783 | 10.633 | 0.15 | 1.39 |
423 | Sunnyvale | 37.37111111111111 | -122.0375 | 39.0 | 128.0 | 140081 | 22.689 | 21.987 | 0.7020000000000001 | 58.765 | 56.946999999999996 | 1.818 | 3.09 |
424 | Susanville | 40.41638888888889 | -120.65305555555557 | 1276.0 | 4186.0 | 17974 | 8.017000000000001 | 7.931 | 0.086 | 20.763 | 20.541 | 0.222 | 1.07 |
425 | SutterCreek | 38.393055555555556 | -120.8025 | 362.0 | 1188.0 | 2501 | 2.5580000000000003 | 2.5580000000000003 | 0.0 | 6.625 | 6.625 | 0.0 | 0.0 |
426 | Taft | 35.1425 | -119.4563888888889 | 291.0 | 955.0 | 9327 | 15.113 | 15.113 | 0.0 | 39.143 | 39.143 | 0.0 | 0.0 |
427 | Tehachapi | 35.132222222222225 | -118.44888888888889 | 1210.0 | 3970.0 | 14414 | 9.971 | 9.874 | 0.09699999999999999 | 25.823 | 25.573 | 0.25 | 0.97 |
428 | Tehama | 40.02444444444444 | -122.12388888888889 | 64.0 | 210.0 | 418 | 0.794 | 0.794 | 0.0 | 2.057 | 2.057 | 0.0 | 0.0 |
429 | Temecula | 33.50333333333333 | -117.1236111111111 | 310.59 | 1019.0 | 105208 | 30.166999999999998 | 30.151 | 0.016 | 78.133 | 78.092 | 0.042 | 0.05 |
430 | TempleCity | 34.10277777777778 | -118.05805555555555 | 122.0 | 400.0 | 35558 | 4.006 | 4.006 | 0.0 | 10.374 | 10.374 | 0.0 | 0.0 |
431 | ThousandOaks | 34.18944444444444 | -118.875 | 270.0 | 886.0 | 128374 | 55.181000000000004 | 55.031000000000006 | 0.15 | 142.918 | 142.53 | 0.387 | 0.27 |
432 | Tiburon | 37.87361111111111 | -122.45666666666666 | 4.0 | 13.0 | 8962 | 13.182 | 4.446000000000001 | 8.736 | 34.14 | 11.515 | 22.625 | 66.27 |
433 | Torrance | 33.834722222222226 | -118.34138888888889 | 27.0 | 89.0 | 147027 | 20.553 | 20.477999999999998 | 0.075 | 53.233000000000004 | 53.038000000000004 | 0.195 | 0.37 |
434 | Tracy | 37.738055555555555 | -121.43388888888889 | 16.0 | 52.0 | 82922 | 22.139 | 22.003 | 0.136 | 57.34 | 56.986999999999995 | 0.35200000000000004 | 0.61 |
435 | Trinidad | 41.05916666666666 | -124.14305555555556 | 53.0 | 174.0 | 367 | 0.6709999999999999 | 0.485 | 0.18600000000000003 | 1.7369999999999999 | 1.255 | 0.48200000000000004 | 27.75 |
436 | Truckee | 39.342222222222226 | -120.20361111111112 | 1773.0 | 5817.0 | 16180 | 33.654 | 32.321999999999996 | 1.3319999999999999 | 87.162 | 83.713 | 3.449 | 3.96 |
437 | Tulare | 36.20666666666667 | -119.3425 | 88.0 | 289.0 | 59278 | 21.016 | 20.930999999999997 | 0.085 | 54.433 | 54.211999999999996 | 0.221 | 0.41 |
438 | Tulelake | 41.95416666666667 | -121.47583333333334 | 1230.0 | 4035.0 | 1010 | 0.41200000000000003 | 0.41 | 0.002 | 1.067 | 1.061 | 0.006 | 0.58 |
439 | Turlock | 37.505833333333335 | -120.84888888888888 | 31.0 | 102.0 | 69733 | 16.928 | 16.928 | 0.0 | 43.843999999999994 | 43.843999999999994 | 0.0 | 0.0 |
440 | Tustin | 33.73972222222222 | -117.81361111111111 | 43.0 | 141.0 | 75540 | 11.082 | 11.082 | 0.0 | 28.701 | 28.701 | 0.0 | 0.0 |
441 | TwentyninePalms | 34.138333333333335 | -116.07249999999999 | 607.0 | 1991.0 | 25768 | 59.143 | 59.143 | 0.0 | 153.179 | 153.179 | 0.0 | 0.0 |
442 | Ukiah | 39.150277777777774 | -123.20777777777778 | 193.0 | 639.0 | 16075 | 4.7219999999999995 | 4.67 | 0.052000000000000005 | 12.232000000000001 | 12.095999999999998 | 0.136 | 1.11 |
443 | UnionCity | 37.58694444444445 | -122.02583333333334 | 72155 | 19.0 | 19.0 | 0.0 | ||||||
444 | Upland | 34.1 | -117.65 | 405.0 | 1328.0 | 73732 | 15.651 | 15.617 | 0.034 | 40.535 | 40.448 | 0.087 | 0.21 |
445 | Vacaville | 38.35388888888889 | -121.97277777777778 | 53.0 | 174.0 | 92428 | 28.585 | 28.373 | 0.212 | 74.03399999999999 | 73.485 | 0.55 | 0.74 |
446 | Vallejo | 38.113055555555555 | -122.23583333333333 | 21.0 | 60.0 | 115942 | 49.5 | 30.6 | 18.8 | 128.3 | 79.4 | 48.8 | 38.0 |
447 | Ventura | 34.275 | -119.22777777777777 | 106433 | 32.095 | 21.655 | 10.44 | 83.124 | 56.085 | 27.039 | 32.53 | ||
448 | Vernon | 34.00111111111111 | -118.21111111111111 | 62.0 | 203.0 | 112 | 5.157 | 4.973 | 0.184 | 13.357000000000001 | 12.88 | 0.47600000000000003 | 3.57 |
449 | Victorville | 34.53611111111111 | -117.28833333333333 | 832.0 | 2726.0 | 120336 | 73.741 | 73.178 | 0.563 | 190.988 | 189.52900000000002 | 1.459 | 0.76 |
450 | VillaPark | 33.816111111111105 | -117.8111111111111 | 104.0 | 341.0 | 5812 | 2.0780000000000003 | 2.0780000000000003 | 0.0 | 5.382999999999999 | 5.382999999999999 | 0.0 | 0.0 |
451 | Visalia | 36.31666666666667 | -119.3 | 101.0 | 331.0 | 124442 | 36.266 | 36.246 | 0.02 | 93.928 | 93.876 | 0.0512 | 0.05 |
452 | Vista | 33.19361111111111 | -117.24111111111111 | 99.0 | 325.0 | 93834 | 18.678 | 18.678 | 0.0 | 48.376999999999995 | 48.376999999999995 | 0.0 | 0.0 |
453 | Walnut | 34.03333333333333 | -117.86666666666666 | 171.0 | 561.0 | 29172 | 8.996 | 8.992 | 0.004 | 23.3 | 23.29 | 0.01 | 0.04 |
454 | WalnutCreek | 37.906388888888884 | -122.065 | 131.0 | 64173 | 19.769000000000002 | 19.757 | 0.012 | 51.201 | 51.169 | 0.031 | 0.06 | |
455 | Wasco | 35.594166666666666 | -119.34083333333332 | 100.0 | 328.0 | 25545 | 9.426 | 9.426 | 0.0 | 24.413 | 24.413 | 0.0 | 0.0 |
456 | Waterford | 37.644999999999996 | -120.7675 | 52.0 | 171.0 | 8456 | 2.369 | 2.3280000000000003 | 0.040999999999999995 | 6.135 | 6.03 | 0.105 | 1.72 |
457 | Watsonville | 36.919999999999995 | -121.76361111111112 | 9.0 | 29.0 | 51199 | 6.7829999999999995 | 6.687 | 0.096 | 17.569000000000003 | 17.319000000000003 | 0.25 | 1.42 |
458 | Weed | 41.424166666666665 | -122.38444444444445 | 1044.0 | 3425.0 | 2967 | 4.795 | 4.79 | 0.005 | 12.417 | 12.405 | 0.012 | 0.1 |
459 | WestCovina | 34.056666666666665 | -117.91861111111112 | 110.0 | 362.0 | 106098 | 16.09 | 16.041 | 0.049 | 41.67100000000001 | 41.545 | 0.126 | 0.3 |
460 | WestHollywood | 34.08777777777778 | -118.37222222222222 | 86.0 | 282.0 | 34650 | 1.8869999999999998 | 1.8869999999999998 | 0.0 | 4.888 | 4.888 | 0.0 | 0.0 |
461 | WestlakeVillage | 34.14194444444444 | -118.81944444444444 | 268.0 | 880.0 | 8270 | 5.5089999999999995 | 5.185 | 0.32 | 14.257 | 13.43 | 0.828 | 5.8 |
462 | Westminster | 33.75138888888889 | -117.99388888888889 | 12.0 | 39.0 | 89701 | 10.049 | 10.049 | 0.0 | 26.026999999999997 | 26.026999999999997 | 0.0 | 0.0 |
463 | Westmorland | 33.03722222222222 | -115.62138888888889 | -164.0 | 2225 | 0.59 | 0.59 | 0.0 | 1.5290000000000001 | 1.5290000000000001 | 0.0 | 0.0 | |
464 | WestSacramento | 38.580555555555556 | -121.53027777777778 | 6.0 | 20.0 | 48744 | 22.846 | 21.425 | 1.421 | 59.172 | 55.49100000000001 | 3.681 | 6.22 |
465 | Wheatland | 39.01 | -121.42305555555556 | 28.0 | 92.0 | 3456 | 1.486 | 1.479 | 0.006999999999999999 | 3.8480000000000003 | 3.8310000000000004 | 0.017 | 0.45 |
466 | Whittier | 33.96555555555556 | -118.02444444444444 | 112.0 | 367.0 | 85331 | 14.7 | 14.7 | 0.016 | 37.0 | 37.0 | 0.040999999999999995 | 0.11 |
467 | Wildomar | 33.603611111111114 | -117.27277777777778 | 387.0 | 1270.0 | 32176 | 23.688000000000002 | 23.688000000000002 | 0.0 | 61.351000000000006 | 61.351000000000006 | 0.0 | 0.0 |
468 | Williams | 39.15472222222222 | -122.14944444444446 | 25.0 | 82.0 | 5123 | 5.444 | 5.444 | 0.0 | 14.100999999999999 | 14.100999999999999 | 0.0 | 0.0 |
469 | Willits | 39.40972222222222 | -123.35555555555555 | 424.0 | 1391.0 | 4888 | 2.803 | 2.798 | 0.005 | 7.26 | 7.247999999999999 | 0.013000000000000001 | 0.17 |
470 | Willows | 39.52444444444444 | -122.19361111111111 | 42.0 | 138.0 | 6166 | 2.873 | 2.847 | 0.026000000000000002 | 7.441 | 7.372999999999999 | 0.068 | 0.92 |
471 | Windsor | 38.54611111111111 | -122.80527777777777 | 36.0 | 118.0 | 26801 | 7.292999999999999 | 7.268 | 0.025 | 18.887999999999998 | 18.824 | 0.064 | 0.34 |
472 | Winters | 38.525 | -121.97083333333333 | 40.0 | 131.0 | 6624 | 2.937 | 2.912 | 0.025 | 7.607 | 7.542999999999999 | 0.065 | 0.85 |
473 | Woodlake | 36.41638888888889 | -119.09944444444444 | 134.0 | 440.0 | 7279 | 2.765 | 2.248 | 0.517 | 7.159 | 5.821000000000001 | 1.338 | 18.69 |
474 | Woodland | 38.67861111111111 | -121.77333333333333 | 21.0 | 69.0 | 55468 | 15.302999999999999 | 15.302999999999999 | 0.0 | 39.634 | 39.634 | 0.0 | 0.0 |
475 | Woodside | 37.420833333333334 | -122.25972222222222 | 117.0 | 384.0 | 5287 | 11.732000000000001 | 11.732000000000001 | 0.0 | 30.386 | 30.386 | 0.0 | 0.0 |
476 | YorbaLinda | 33.888551 | -117.813231 | 82.3 | 270.0 | 65237 | 20.018 | 19.483 | 0.535 | 51.847 | 50.46 | 1.3869999999999998 | 2.67 |
477 | Yountville | 38.403055555555554 | -122.36222222222221 | 30.0 | 98.0 | 2933 | 1.531 | 1.531 | 0.0 | 3.966 | 3.966 | 0.0 | 0.0 |
478 | Yreka | 41.72666666666667 | -122.6375 | 787.0 | 2582.0 | 7765 | 10.052999999999999 | 9.98 | 0.073 | 26.035999999999998 | 25.846999999999998 | 0.188 | 0.72 |
479 | YubaCity | 39.13472222222222 | -121.6261111111111 | 18.0 | 59.0 | 64925 | 14.655999999999999 | 14.578 | 0.078 | 37.959 | 37.758 | 0.201 | 0.53 |
480 | Yucaipa | 34.030277777777776 | -117.04861111111111 | 798.0 | 2618.0 | 51367 | 27.893 | 27.888 | 0.005 | 72.244 | 72.23100000000001 | 0.013000000000000001 | 0.02 |
481 | YuccaValley | 34.13333333333333 | -116.41666666666667 | 1027.0 | 3369.0 | 20700 | 40.015 | 40.015 | 0.0 | 103.639 | 103.639 | 0.0 | 0.0 |